Regex Pattern Matching Phone Numbers
The first step in performing a Regex check with Python is to create a regex pattern that matches phone numbers. Then we will use the built-in Python Regex module to test the string that a user provides against the Regex pattern we have created.
Common Problems With Phone Number Validation
Similar to matching email addresses, matching phone numbers with Regex is not recommended in production. Phone number validation with a regular expression is tricky, particularly when your app must handle international numbers.

Number Length
As mentioned, valid US phone numbers are 10 digits long (when the area code is included and the +1 is not), but a valid Mexican phone number is 12 digits long. In some countries, phone numbers are longer. The maximum length for a international phone number is 15 digits, according to the International Telecommunication Union (ITU.)
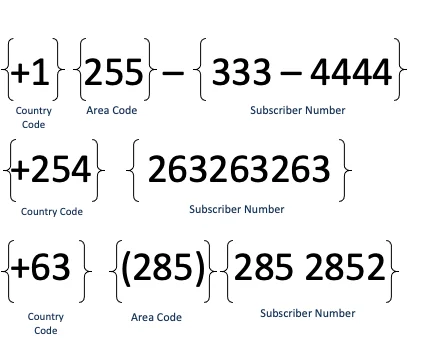
Delimiters
Delimiters (the characters that come between the numbers in phone numbers) are also different all over the world. Some countries use the em-dash (-), while others use a period or dot (.) and still, others use parentheses, tildes, or other separators. The way numbers are grouped together in phone numbers also differs from country to country.
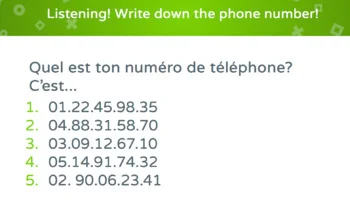
User Error
All these differences lead to a large margin for user error. Some users will try to include dashes in their phone numbers while others will not. Some users will include country codes. Some will include spaces whereas others will not. Some users might omit the area code or the +1 from a US number.

The Problem With Using Regex to Match Phone Numbers
All that being said, you will almost immediately come up against these problems when using a Regex pattern to match phone numbers because there are many different ways to format a phone number. Writing a single specific pattern to capture all the characters and possibilities is impossible.
For this reason, we don't recommend relying solely on Regex phone number pattern matching to handle parsing phone numbers and performing your phone number validation. Using a Regular expression in tandem with a dedicated third-party service is the most robust way to check for a valid number.
Parsing Phone Numbers
One recommended method for dealing with international phone numbers and other phone number matching issues is to strip all the characters that aren't numeric digits from the string before you begin.
Unfortunately, this method doesn't work very well either, as all you are left with after you remove anything that isn't a numeric digit is a string of numbers that could be between 7 and 15 characters long. This doesn't give you much to work with to determine whether or not the string is a valid number.
A Good Regex Pattern for Matching Phone Numbers
Let's take a look at some basic regular expression syntax that matches US phone numbers. Keep in mind that this pattern can only be relied upon to match US phone numbers. Here is the pattern:
This specific pattern will match US phone numbers in the following formats:
Let's look at how we would use this regular expression in our Python code.
Here, we imported the Python re module, which provides methods for working with regular expressions. We created a variable to hold our phone number pattern, and another variable to hold our phone number.
Next, we used the re.search function to search the input string for a match to the regular expression. The re.search function searches the input string from the beginning of the string and creates a Match object if a matching substring is found.
The result of printing the match object will look like this:
The Match object contains information about the search and the result. The span field indicates the positions in the string where the match started and ended. The match field indicates the match that was found. If the match was a substring of the input string, a group field will indicate where the matched group was found.
If no match is found, re.search will return null. You can therefore examine the result of the re.search function to determine if input phone numbers are valid: a valid number will return a match, while an invalid phone number will return null.
Validating Phone Numbers With an API
Short of sending an SMS to a number to determine its validity (which you should always do as part of your phone number verification process) the best way to check for valid or invalid phone numbers is to use a dedicated third-party library or API.
One such service is AbstractAPI's Free Phone Number Verification API. This API provides an endpoint to which you can send a phone number string and receive a response telling you whether or not the string is a valid phone number.
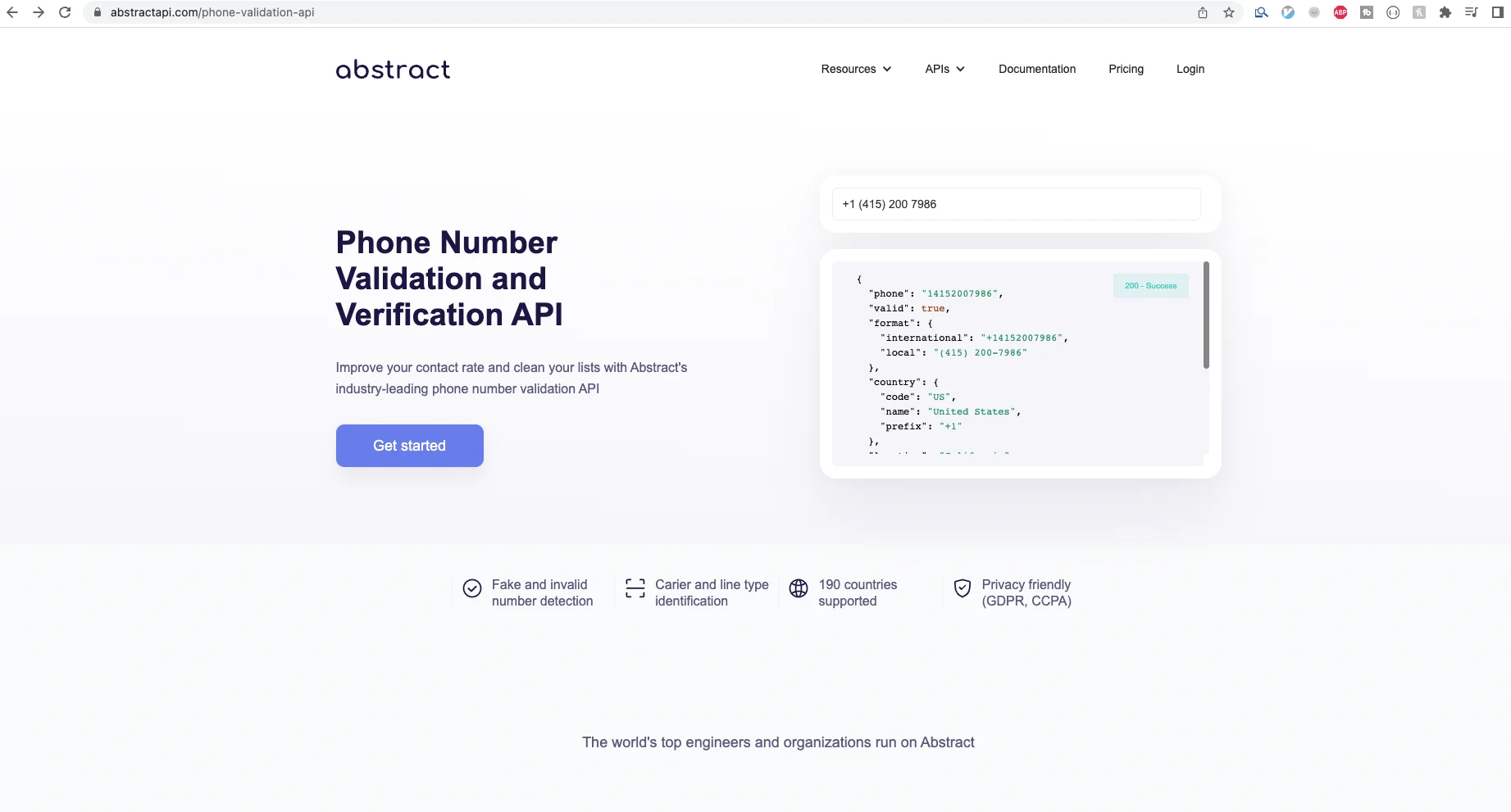
AbstractAPI performs several checks to determine the validity of the number. First, they check the number against a regular expression. Next, they verify the number against a regularly updated database of phone numbers to determine whether the number is a disposable number, VOIP, a free phone number, or another type of low-quality number.
The API returns a JSON object a validity boolean and information about the number's carrier and location information.
Let's look at how we can use AbstractAPI's Free Phone Validation endpoint to check the validity of a provided number.
Acquire an API Key
Go to the Phone Validation API Get Started page and click the blue “Get Started” button.
You’ll need to sign up with an email address and password to acquire an API key. If you already have an AbstractAPI account, you'll be asked to log in. Next, you’ll land on the API’s homepage, where you’ll see options for documentation, pricing, and support.
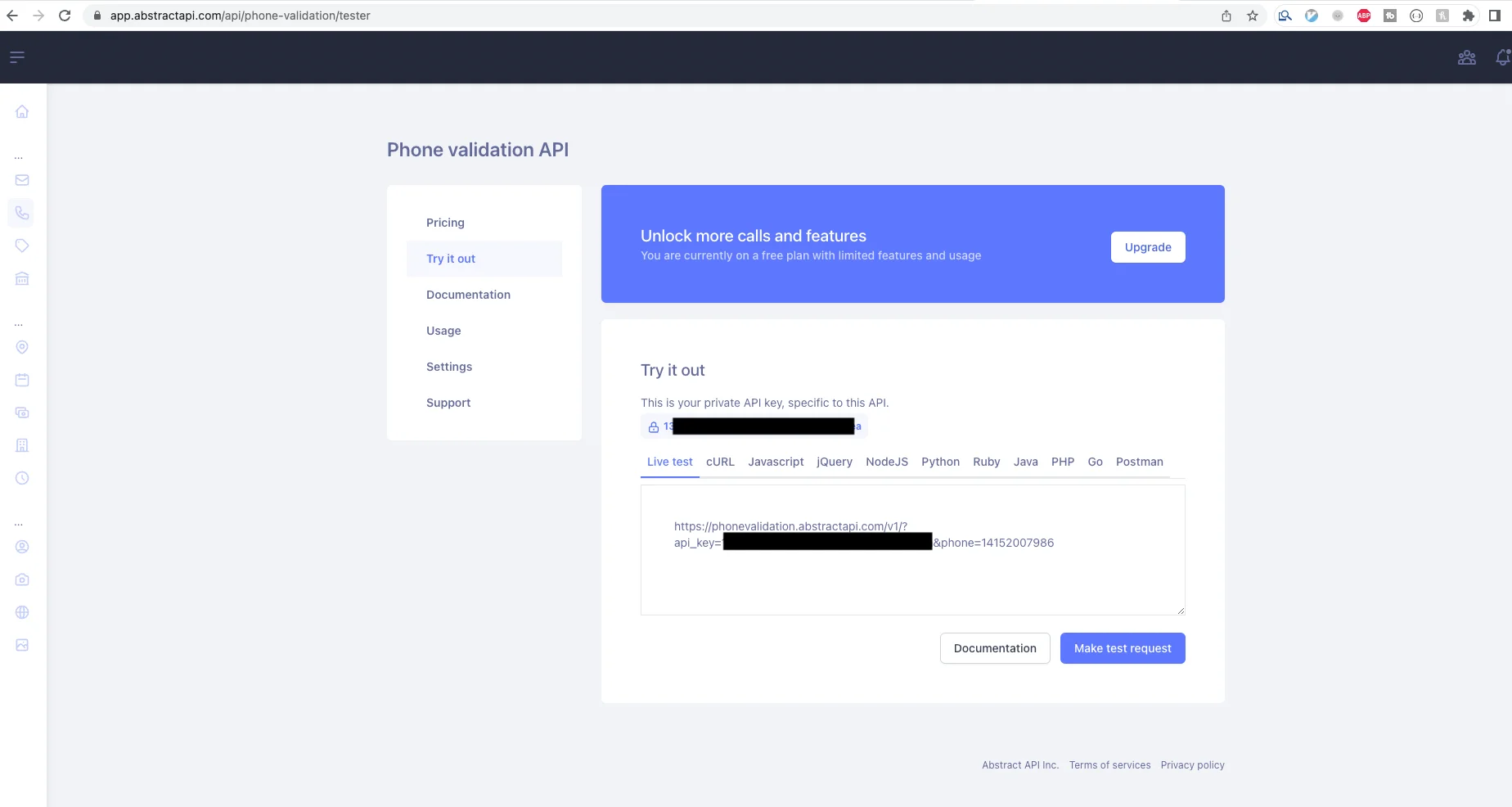
Look for your API key, which will be listed on this page. Every Abstract API has a unique key, so even if you’ve used a different Abstract API endpoint before, this key will be unique.
Send a Request to the API
We'll use the built-in Python requests module to send a POST request to the API endpoint with the number for validation.
The response sent back by the API will look something like this:
From here, all we need to do is extract the valid field from the response object and use its value to determine the validity of the number.
The best part about the AbstractAPI endpoint is that it works across different programming languages. Simply toggle the language choice in the sandbox on your dashboard to see options for Javascript, Ruby, Node, Java, and more.
Conclusion
In this tutorial, we looked at how to use Regex and Python to determine the validity of phone numbers. We also discussed why relying on Regex patterns for verification of your phone numbers isn't a good idea in production. We then looked at AbstractAPI's Free Phone Validation endpoint as an alternative to regular expressions.
FAQs
How do you write Regex in a phone number in Python?
Matching phone numbers, like matching email addresses, is tricky with regular expressions. There is no specific Regex pattern that will match all international numbers. Phone numbers all over the world have different formats, delimiters, grouping mechanisms, and lengths.
For that reason, it's recommended that you use a library or service to validate phone numbers in your web or mobile application, and don't rely solely on a regular expression.
How do you validate a phone number in Python?
There are several ways to validate phone numbers in Python. You can check them against a regular expression to find out whether the phone numbers are properly formatted (be aware that this is error-prone and not recommended for production apps.) You can use a library like phonenumbers. Finally, you can rely on a third-party API or service like AbstractAPI to validate phone numbers for you and return a JSON response with the result.
What is phone numbers library in Python?
The phone numbers library is an open-source Python module that facilitates cleaning, parsing, examining, and validating phone numbers. It is a Python port of the Google libphonenumbers library.