Basics of 10-Digit Phone Number Validation
For the purposes of this article, we’ll assume that the phone numbers we’re dealing with are standard American 10-digit phone numbers. Validating international phone numbers is a separate concern that presents its own set of challenges because of the different phone number formats around the world.
Even when we constrain our tutorial to 10-digit mobile numbers, however, we still face challenges. Users cannot be relied on to fill out an input field correctly. They frequently mistype things, enter things in unexpected formats, and forget things.
That’s why it’s so important to validate your phone number input.
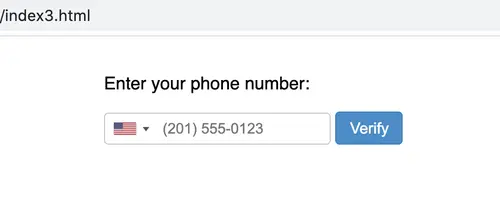
10-Digit Phone Number Validation in HTML
The first thing you should do in your web forms is make inputting information as clear and straightforward as possible. Some things you can build into your web form to make things easy for users include the following:
- Have a dropdown to select the country code so the user doesn’t try to input it into the phone number input.
- Use placeholder text to demonstrate the exact format you want users to use when inputting their phone number
- Use geolocation to detect the country your user is in, and autofill parts of the form (like the country code) for them
- Strip out special characters like dashes and parentheses before you send the phone number to any kind of back end for processing.

HTML5 Input Validation
Once you have your form set up correctly, you can use HTML5 to do form validation without having to use Javascript, PHP, Python, C#, or any other programming language. This is the easiest way to validate form inputs in your code.
First of all, use the tel input type to tell you the HTML file that this input should accept a phone number:
You can also add the required attribute if this information is something your user needs to provide before they can proceed.
The HTML will handle showing some error states to handle missing information all on its own.

Regex for Phone Number Validation
You can also use the HTML5 pattern attribute and Regex to do some basic validation of the phone number format. Regex (regular expressions) allows you to define a pattern and then compare user input to the pattern to make sure it matches.
There is no “one” pattern to validate a phone number using Regex. You will have to search for some examples of common patterns and decide which one works for you. You can use a tool like https://regexr.com/ to create and test your Regex patterns.
Implementing 10-Digit Phone Number Validation with Regex
A regular expression for 10-digit mobile number validation might look something like this:
This assumes you want your user to input the number in the xxx-xxx-xxxx format. If you’d rather have your user input the number without dashes or other special characters, you could use a regular expression like this:
This will look for numbers formatted like xxxxxxxxxx.
Once you have decided on the regex pattern you want to use for validation, apply it to your HTML5 form input:
APIs for Phone Number Validation
HTML5 can do some very basic validation, and Regex works fine for simple use cases, but neither of them should be relied upon alone to perform validation in a production app. Regex is notoriously unreliable and it’s impossible to capture every type of valid phone number or user error.
A better way to validate phone numbers is to use a dedicated phone number verification API. Let’s take a look at how to use one such API - the AbstractAPI Free Phone Validation API - to validate a 10-digit number.
Acquire an API Key
Go to the API documentation page in your browser. Click the “Get Started” button.

You’ll be asked to input your email and create a password to create a free account, and then you’ll land on the API dashboard where you’ll see your API key.
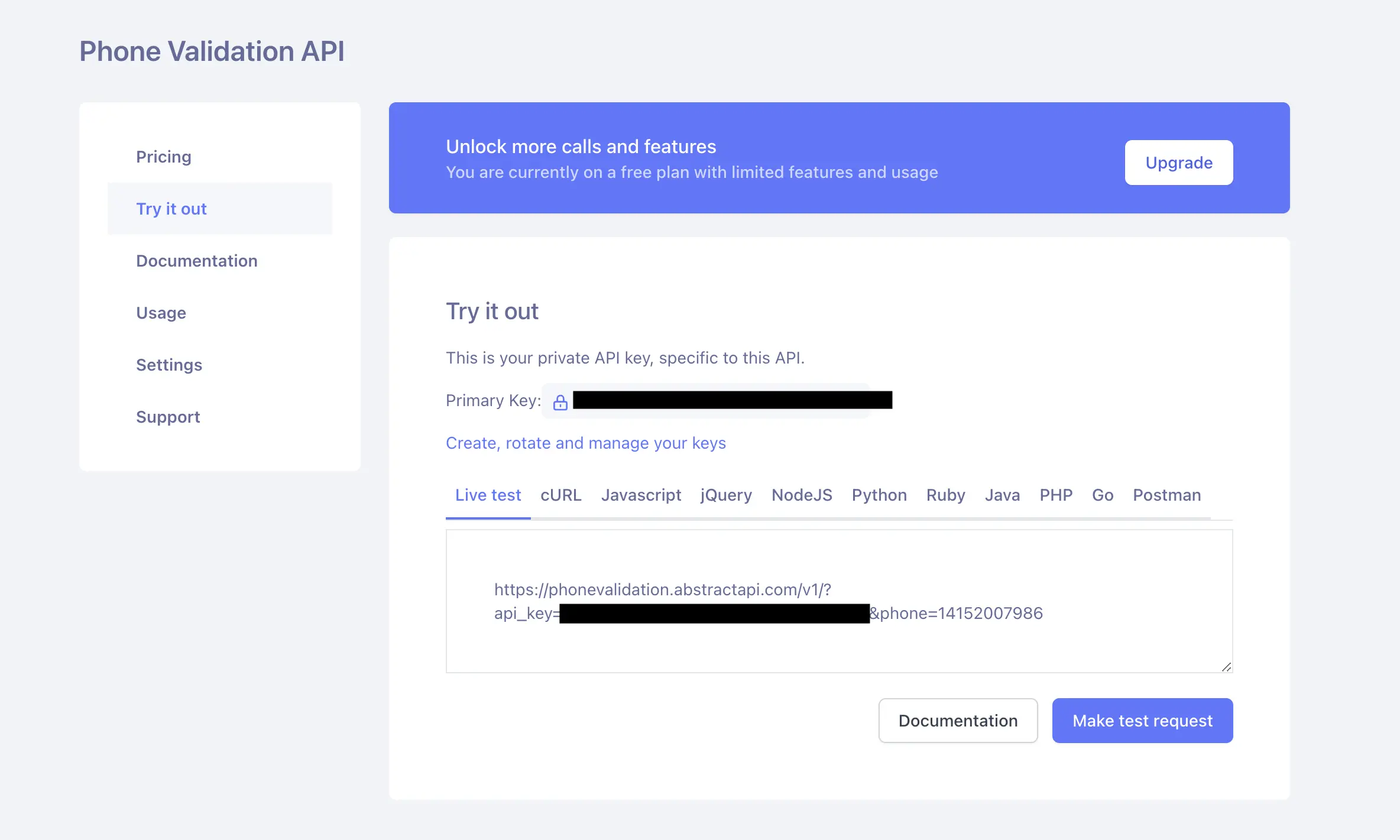
Make a Test API Request
Use the provided “Make test request” button to send your first request to the API. Make sure that the “Live Test” tab is selected above the provided input box. The URL inside the box includes a query string that contains your API key and an example phone number.
Click the “Make test request” button. You should receive a 200 status and a JSON response. Let’s take a look at the response object:
This is all of the information that will be returned to you about a phone number by the free version of the API. To get more detailed information, you can upgrade to a paid plan.
Make a Real API Request
Abstract provides code snippets for all the major programming languages. Simply choose the language you need from the tab selection above the textbox and click the blue “Copy Code” button, or highlight and right-click to copy the provided code snippet

Paste the snippet into your JavaScript, Python, or other language file to send the request from your own app.
Common Mistakes and How to Avoid Them
Over-reliance on front-end validation
Don’t solely rely on HTML and Regex to validate your phone numbers. You should use a combination of these methods plus a reliable API to perform comprehensive checks.
Not accounting for different formats
Every user will try to input a number differently - with dashes, spaces, dots, parentheses, with area code, without area code, with country code, etc. Do as much work as possible for your users and make it very clear and easy for them to input their numbers.
Overcomplicating regex patterns
The best Regex patterns are as simple as possible. If all you need to do is check that a user has entered 10 digits, make sure that’s all your Regex is checking for. And remember: Regex is not a reliable way on its own to validate inputs.
Conclusion
It’s very easy to validate a phone number using simple HTML and Regex - however, for a more robust solution, it’s recommended that you use a dedicated API like AbstractAPI’s Free Phone Number Validation API.
Remember to make your HTML forms clear and easy to use, and do as much work as possible for your users up front to ensure they get their information entered smoothly and efficiently.