API-Based Validation
While regular expressions can catch incorrect email formats, they can’t confirm if an email address is real or if its domain is active. For deeper and more accurate email validation, using an API like AbstractAPI’s Email Validation API is highly recommended.
This approach checks for domain activity and existence, offering a reliable solution for applications that require accurate user data.
By integrating an API, you can go beyond basic formatting checks and validate emails in real time. This level of validation is especially useful for applications that rely on authentic user email addresses for:
- Secure communications.
- Account verification.
- Email notifications.
Let’s see how to set up a basic API call in Rails using Net::HTTP and URI:
require 'uri'
require 'net/http'
def validate_email(email)
uri = URI("https://emailvalidation.abstractapi.com/v1/?api_key=your_api_key&email=#{email}")
response = Net::HTTP.get(uri)
JSON.parse(response)["is_valid_format"]["value"]
end
In this example, the validate_email method sends a request to the AbstractAPI endpoint, where it checks if the email format is valid if the domain exists, and if it’s active. Replace "your_api_key" with your actual API key to use this service.
Why Use API-Based Validation?
This API-based approach is a powerful option for Rails applications that need precise validation because it ensures:
- Higher Data Quality (reduces the chances of invalid or fake email entries, leading to a cleaner user database).
- Better Security (by confirming that email domains are active, the application can avoid spam, fake accounts, or potential security vulnerabilities).
- Improved User Experience (users are notified immediately if there is an issue with their email).
So, using an API like AbstractAPI offers an efficient solution to verify both email syntax and existence. This approach improves the accuracy of user data, minimizes fake or inactive entries, and contributes to a more reliable experience for users.
Gem-Based Validation
In Rails, using gems for email validation adds functionality with minimal setup. Gems are packages that extend Rails applications, allowing developers to implement complex validation features easily. One recommended gem is valid_email2.
Recommended Gem: valid_email2
Let’s see what it is good for!
The valid_email2 gem goes beyond basic format checks by verifying MX (Mail Exchange) records to ensure the domain can receive emails. It also filters out disposable email addresses often used for spam, which helps maintain data quality.
Installation and Setup
Add to Gemfile: Add the gem to your Gemfile and install it.
gem 'valid_email2'
Install and Configure: Run bundle install and set up validation in the model.
class User < ApplicationRecord
validates :email, presence: true, email: true
end
This setup checks that the email is present, correctly formatted, and from a valid domain.
Benefits of valid_email2
- Better Data Quality: Filters out temporary emails, reducing spam and fake registrations.
- Increased Security: MX checks verify that domains are capable of receiving emails.
- Easy Setup: valid_email2 simplifies complex validations, making it beginner-friendly.
For Rails applications needing accurate email validation, valid_email2 offers a reliable, secure, and efficient solution.
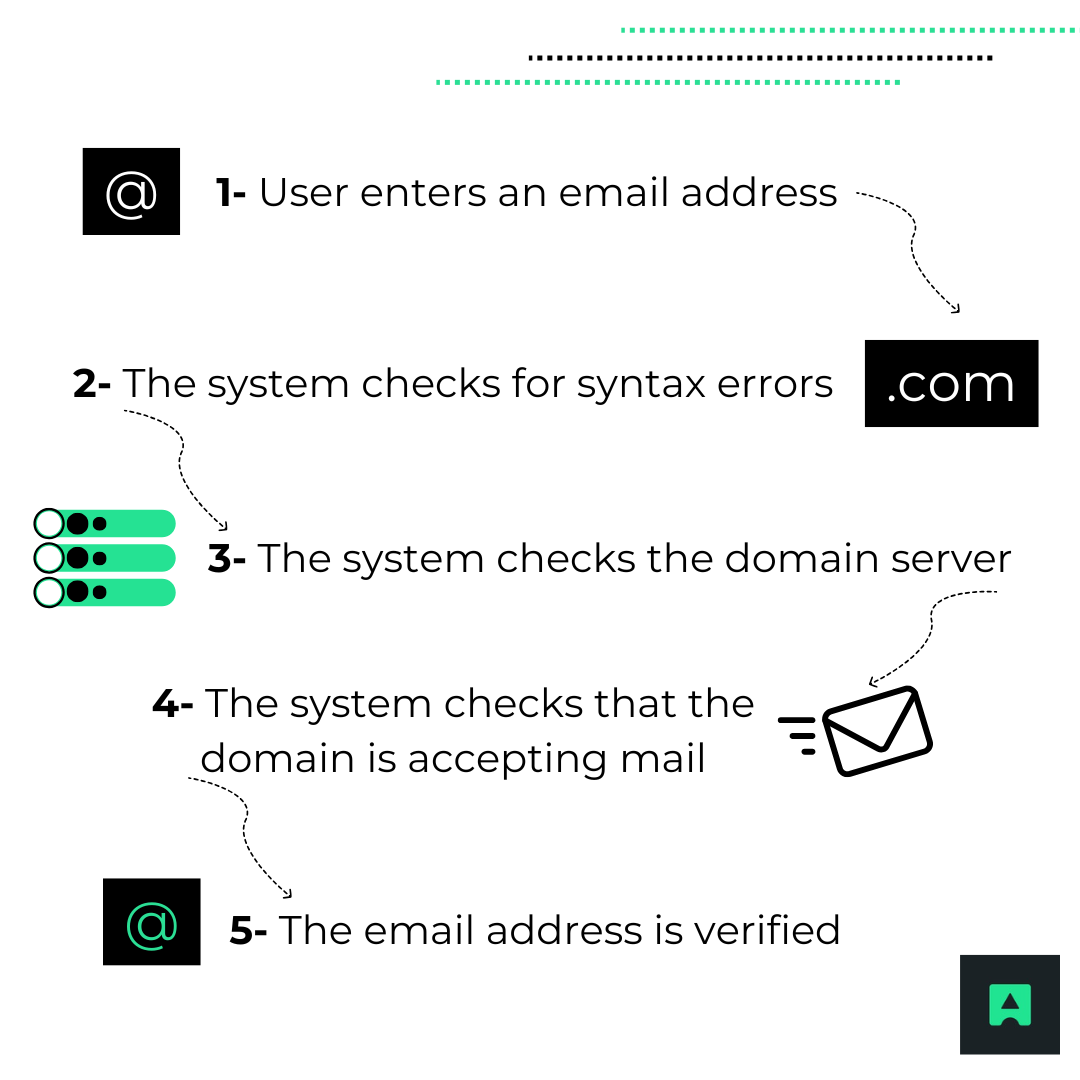
How to Send Confirmation Email in Rails?
Adding a confirmation email in a Rails application provides an essential layer of user verification, helping to ensure that each user’s email address is valid and belongs to them. It reduces the risk of spam accounts and increases the reliability of your user base.
Using Action Mailer
Rails has a built-in tool for sending emails called Action Mailer. It makes it easy to configure and send emails, such as account confirmations, password resets, and notifications.
Here is how to set up a simple confirmation email!
1- Generate the Mailer: Start by generating a mailer for user confirmations.
rails generate mailer UserMailer
Also, this creates a mailer class where you can define different email methods, such as a confirmation email for new users.
2- Configure the Mailer: Next, open the UserMailer class and define a confirmation_email method. This - method will structure the email with the user’s details and a subject line.
class UserMailer < ApplicationMailer
def confirmation_email(user)
@user = user
mail(to: @user.email, subject: 'Confirm Your Account')
end
end
In this setup, @user represents the new user’s data, and the mail method specifies the recipient’s email and the subject. The email content can be customized by creating a corresponding view template for the confirmation_email method, allowing you to design a professional-looking message.
3- Trigger the Email: To send the confirmation email when a user signs up, you can trigger it in the controller or model where the user is created.
For example:
UserMailer.confirmation_email(@user).deliver_now
This will send the email immediately, ensuring the user receives it right after registration.
Benefits of Sending Confirmation Emails
- Improved Data Quality: Confirms the user’s email address, reducing errors and spam accounts.
- Enhanced Security: Adds an extra step in verifying user identity.
- Professional Experience: Provides users with a clear, trustworthy registration process.
Setting up confirmation emails in Rails with ActionMailer improves the user experience, strengthens data accuracy, and adds a layer of security to your application.
Active Record Validation
Rails Active Record includes a range of built-in validators that make email validation easy and effective. With these validators, you can quickly ensure that user-submitted email addresses meet your application's standards.
Basic Active Record Validations
To validate emails, you can combine the presence, uniqueness, and format validators for a solid, straightforward approach:
class User < ApplicationRecord
validates :email, presence: true, uniqueness: true, format: { with: URI::MailTo::EMAIL_REGEXP }
end
Side note: This setup ensures that the email field is not empty, is unique in the database, and follows a valid email format.
Advanced Validation with Custom Validators
For special cases, Active Record also allows you to define custom validators. For example, you could create a custom rule allowing only email addresses from specific corporate domains, adding flexibility for applications with unique requirements.
Additional Tips
Combining validation and email confirmation is a powerful approach to enhance both security and user experience in Rails applications. By implementing both techniques, you ensure that each email address not only meets validation standards but also belongs to an actual, engaged user who confirms their identity. This two-step process can significantly reduce the chances of fake or inactive accounts, helping to maintain a cleaner and more reliable user database.
For example, using Active Record validations ensures that users provide correctly formatted, unique email addresses, while Action Mailer allows you to send confirmation emails that prompt users to verify their email ownership. Together, these tools make the registration process more secure and trustworthy for users.
For further guidance, explore the following Rails documentation resources:
- Action Mailer Documentation: A guide on setting up and sending emails in Rails.
- Active Record Validations Documentation: A comprehensive overview of the validation methods available in Rails.