Vue IP Geolocation
Today, we're going to use Vue to capture a user’s geolocation data through their IP address and display that data to the user. Geolocation or the process of determining a device’s location through things like IP address or GPS is incredibly useful for doing things like displaying localized content (for example, language translations and currency conversions) and securing user interactions in your app.
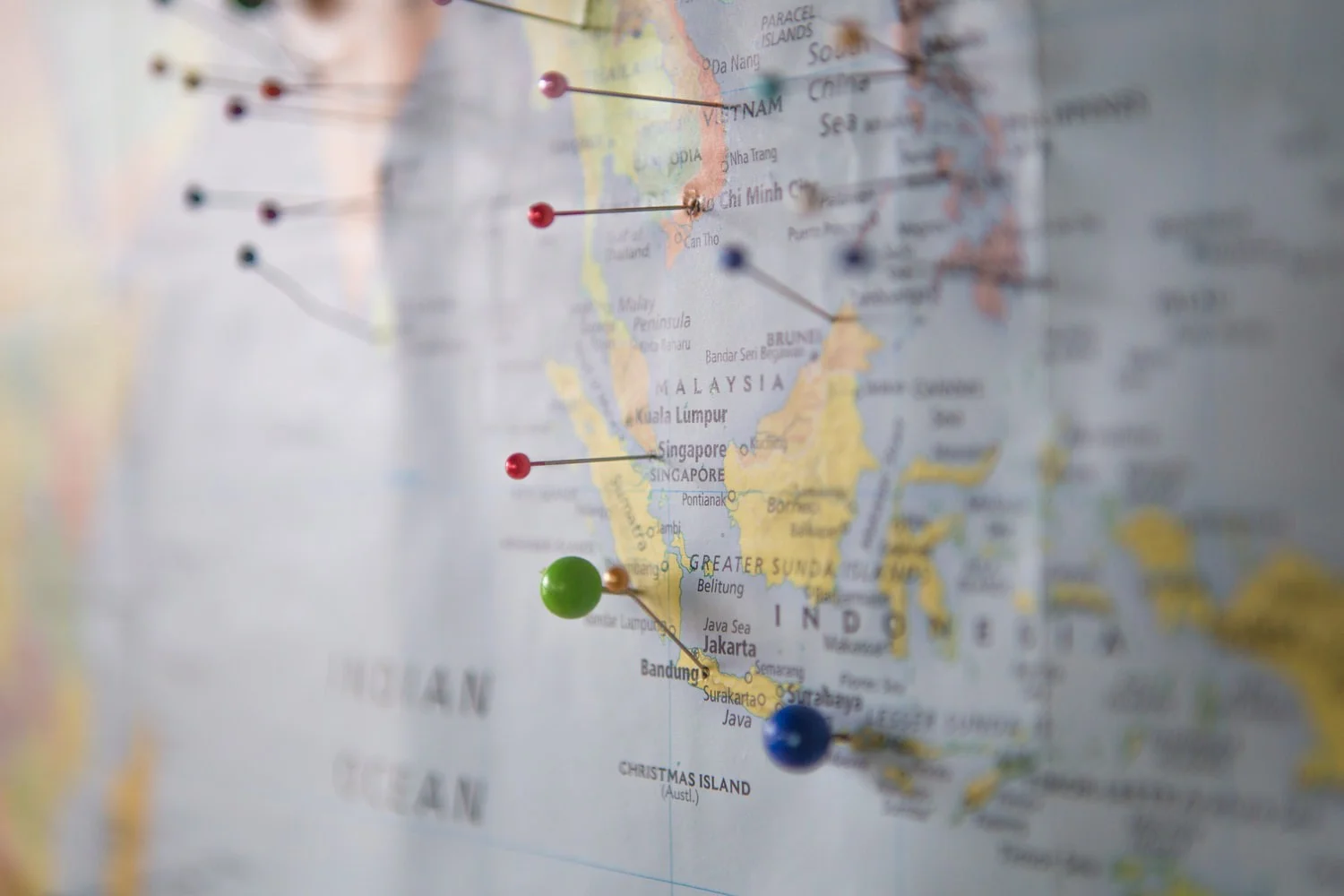
This tutorial will assume at least some understanding of Vue, but it can be completed by beginners. We’ll go through basic Vue installation and component setup, and we’ll build a simple page that shows the user their current location.
Get Started With Vue
The easiest way to get started with Vue is to read the docs. Vue does a lot of cool stuff that we won't have time to cover in this tutorial. Here, we’ll quickly outline the basic process for getting up and running with Vue..
Vue Quick Start
To get started with Vue, run the following command.
Notice that there is no vue cli. This takes you through the Vue installation process and will ask if you want to install things like Typescript, ESLint, Cypress to set up automated testing, and Pinia for managing state. None of these things are necessary for this tutorial, so you can select "No" for them unless you’d like to use Typescript or linting, or set up automated testing. Name the app something like "vue-geolocation".
A new folder will be created with the name you gave to the Vue setup wizard. Navigate to the project directory root, install the dependencies, and start the development server.
By default, Vue runs on port 5173. Visit http://localhost:5173 in the browser to see your new Vue app. There will be a lot of boilerplate that we won’t use.

Create a Basic Vue Component
Open up the project folder in your code editor (VS Code is a great choice for working with Vue.) Take a look at App.vue, which is the main app file and handles mounting the root app for your project.
Currently, there are two components that have been imported into the Vue file: a <Welcome> component, and a <HelloWorld> component. These components are defined inside src/components. They are boilerplate components provided by Vue to give you something to work with right away.
Usually, you would want to create discrete, meaningful components for each piece of logic or part of the UI in your app. For now, however, since our project will be so small and simple, we'll simply delete the existing components and build everything directly in the App.vue file.
Create a Vue SFC
A Vue SFC is a Single-File Component. It has a .vue extension and allows you to write Javascript, HTML, and CSS in the same file. This can make organizing your code easier and keep logic for specific components well-contained.
Delete everything from App.vue and add the following.
Here, inside the <script> tag, we've created a default component export, similar to what we would do if we were using React. Vue uses the data value to bind data in the Javascript component to the HTML template. Inside the <template> tag, we've created a simple <h2> and <p>. We’ve rendered the value of the city, country, and region data inside the <p> tag using handlebar syntax.
Take a look at http://localhost:5173 to see the new app. It doesn’t look very exciting yet—we are missing our key pieces of data.
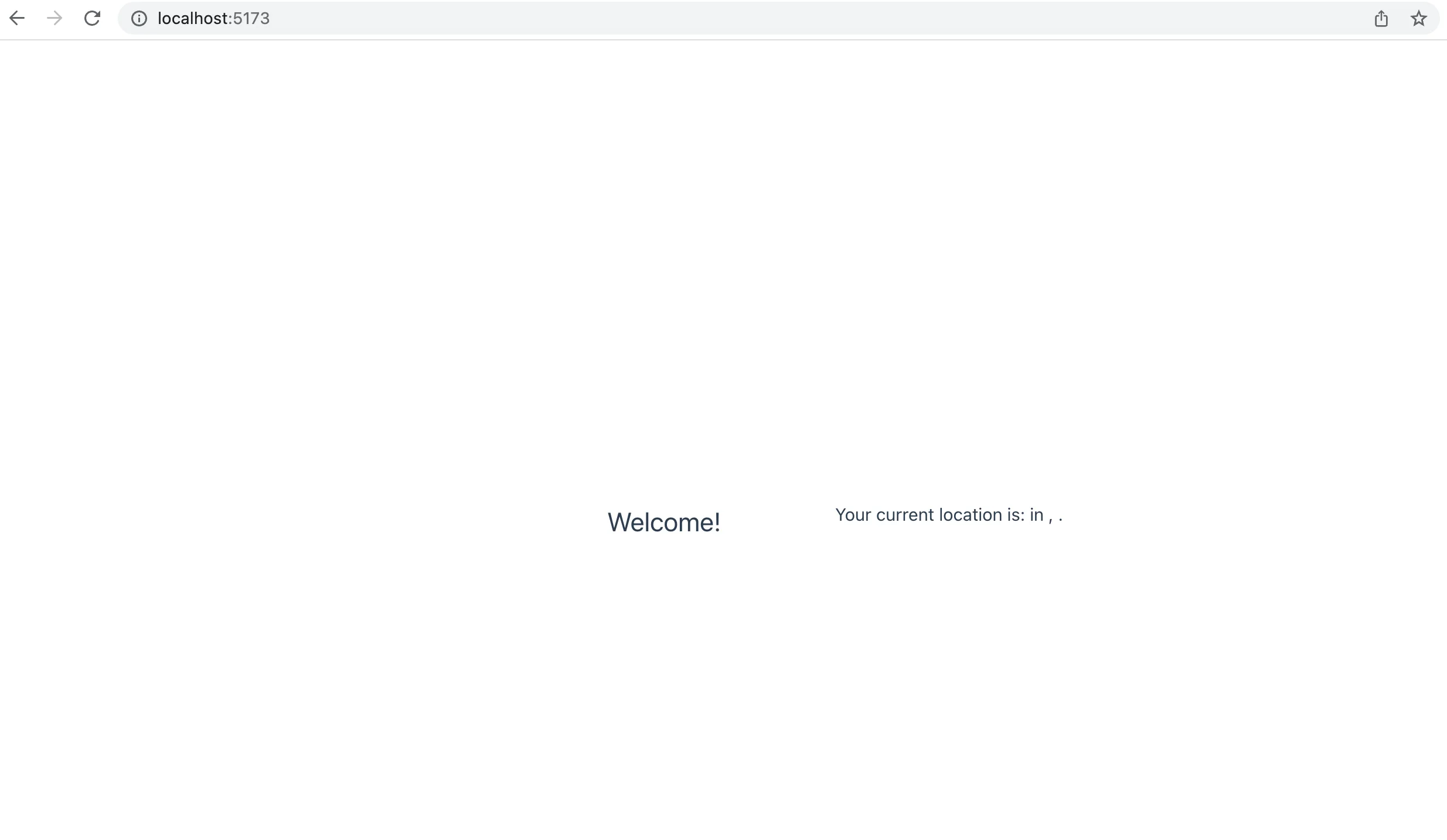
Let’s send a request to the AbstractAPI Free IP Geolocation endpoint to get back some information about our location.
Get User Location Through IP
One of the easiest ways to get location data is by looking up the user’s IP address. Many free services will return geolocation data about a device based on its IP address. The AbstractAPI Free IP Geolocation endpoint is one of those services.
When you send a request to the API, you can optionally include an IP address parameter. The API will send back a JSON object with detailed information about the IP address’s current location, including city, state, country, zip code, currency, predicted language, and carrier and device information.
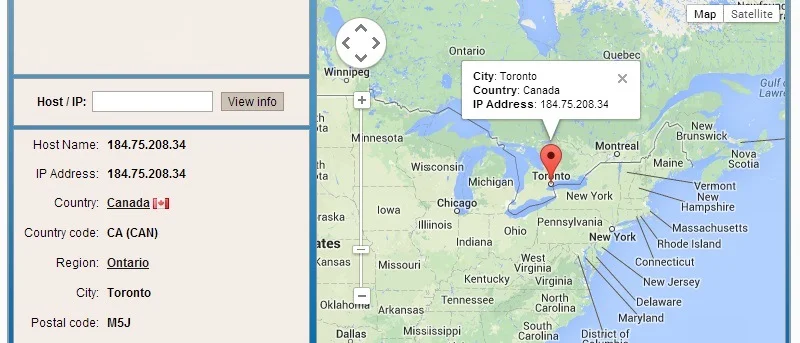
If you do not include the optional IP address parameter, the API will default to sending back information for the IP address of the device that made the initial request. Since we’ll be sending our request from the browser, we can use this method and not include an IP address. This makes our job easier—we don’t need to know the user’s IP address since AbstractAPI will get that information for us.
If we were sending the request from a server, we would need to capture the user’s IP address and pass it along, since otherwise, AbstractAPI would default to using our server’s IP address for the geolocation.
Get Started With the Abstract API
In order to use the API, you'll need to sign up and get an API key. You can sign up for free and the free tier is enough to use for testing and developing an app. If you decide you need more requests than the free tier provides, there are many affordable pricing tiers.
Navigate to the API home page and click "Get Started"
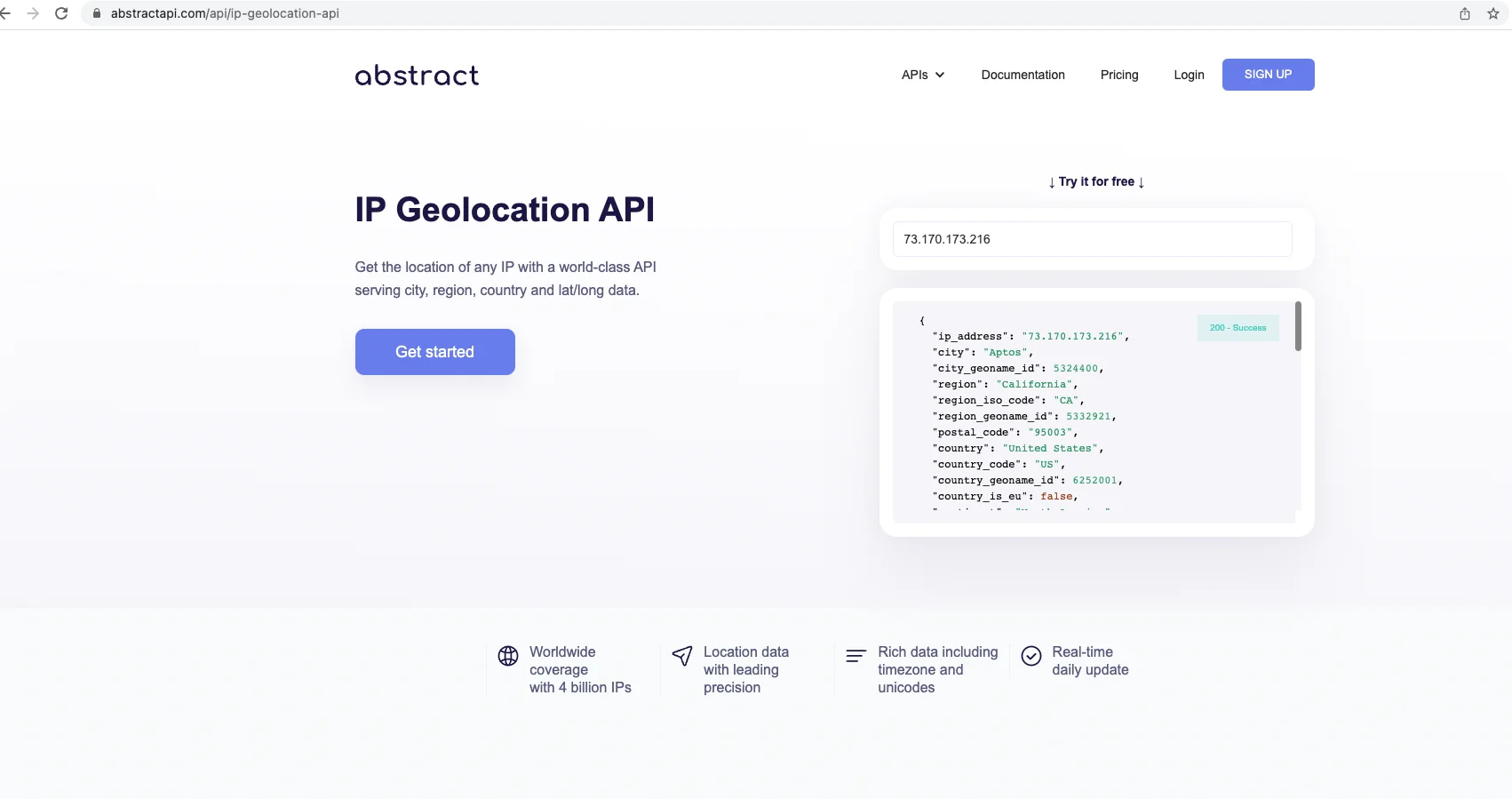
You will be asked to provide an email address and password. Once you've signed up, you'll land on the geolocation API dashboard, where you'll see your API key. Copy the API key and the API endpoint’s URL as we will use them in a moment in our app.
Send a Request to the API
We’re going to write a function called getGeolocationInformation inside our App.vue SFC. The way you create and register methods with your Vue component is by adding them to the methods object on the component export. The methods field is like the data field except that it defines the methods that the component will use to work with data.
Create the method field and add the function. It should look like this.
Now let’s write the code that will send the request to the API and handle the response. Note that we made the getGeolocationInformation method async because we will be making a network request.
Right now, we’re just logging the response. The response from the API will look something like this:
We can easily pull the city, country, and region values out of this response and render them in our app.
Now, we just need to tell the app to call our getGeolocationInformation function when it first loads.
Vue uses lifecycle hooks similar to React in order to determine when certain things should happen in our app.
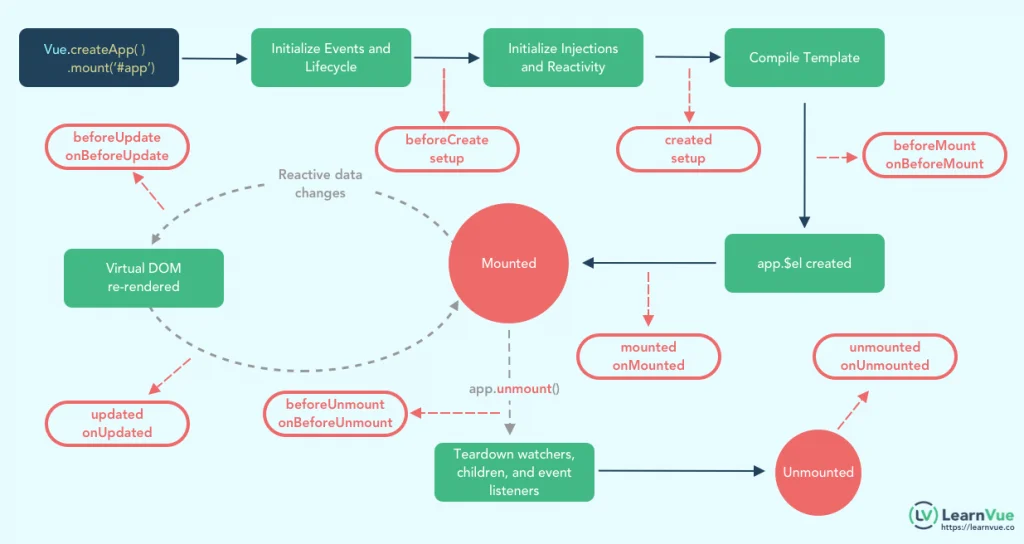
We can use the mounted hook to call our geolocation function when the component mounts.
The request will be made to the API, our information will be returned, and our data will be displayed to the user.
Let’s take a look at our complete App.vue SFC file.
Head back over to localhost:5173 to check out the results in the browser.

Note: identifying location information has been redacted.
Error Handling
In case something goes wrong while we’re making our network request, we should include some error handling to display a special error message to the user.

Create a new data value called errorMessage. Render the value inside a <p> tag just below the existing <p> tag. Let's give the new p tag a red color to show that it's an error.
Next, update the getGeolocationInformation function to show a special error message when the request fails, and remove the message when the request is successful. We’ll wrap the request in a try/catch block.
To test this, you could return to localhost and disconnect from your WiFi. When the app tries to send the request, it will fail, and you should see a red error message.
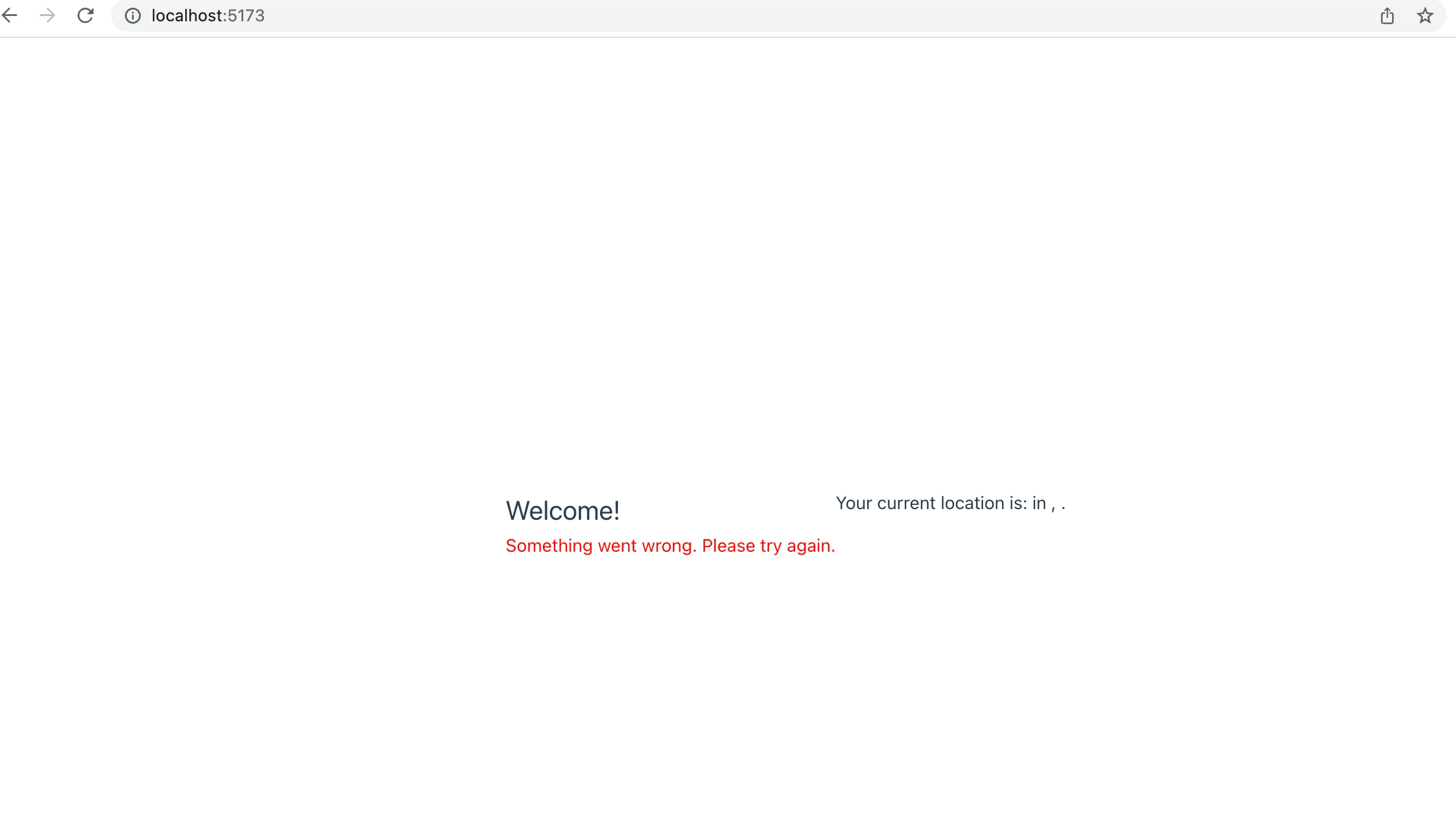
Conclusion
In this tutorial, we learned how to create a basic Vue app that renders user location data. To get the user’s location, we sent the IP address of the user’s device to the AbstractAPI Free IP Geolocation endpoint and got back a JSON object with information about the IP address’s current location.

The next step would be to decide how you want to use the user’s geolocation data. You might want to send the location data to your server so that the server can send back localized or translated content. You may want to display a different currency if the device is in a foreign country. You may want to render the user’s coordinates on a map. There are many things you can do with geolocation data.
FAQs
How Do I Get Geolocation on VueJS?
There are a few ways of doing geolocation in a VueJS app. One of the easiest ways is to do Vue browser geolocation: use the browser’s built-in navigator.geolocation API. This gives you access to the device’s latitude and longitude coordinates. There is a package called vue-browser-geolocation that provides a simple geolocation plugin wrapper for this API along with error-handling, and watchers. Read the package's geolocation docs for more information.
If you want more information than Vue browser geolocation can provide (i.e. more than just a set of coordinate points), send the user’s IP address to a third-party API like AbstractAPI’s Free IP Geolocation endpoint to get back detailed information including city, region, country, currency, language, and latitude and longitude coordinates for a particular IP address.
What Is Navigator Geolocation?
Navigator is a built-in browser API that gives apps running in the browser access to information about the user’s device. The navigator.geolocation API returns a geolocation object that contains a set of latitude and longitude coordinate points for the device’s current location, as well as a timestamp of when those points were read.
Read the navigator geolocation docs for more information.
How Do You Call a Function on Page-Load in Vue?
Vue provides lifecycle hooks similar to React for calling functions at certain times during the component’s lifecycle. We can call a function on page load by calling it inside either the beforeMount hook, the mounted hook, or the created hook.