Geolocate an IP address using an IP address database
An easy way to geolocate an IP address is to use an online geolocation database like Geolocation DB. Geolocation DB is free-to-use and doesn't require an API key. It stores an impressive database of IP addresses obtained from Internet service providers.
You can query the Geolocation DB database to geolocate the IP address 147.229.2.90 using the following code:
For the given IP address, this query returns the following data:
Drawbacks to this approach
Because Geolocation DB is free to use, it does not necessarily have the most accurate or up-to-date data. There also is no way to confirm the validity of the IP address locations.
Additionally, this approach provides bare-bones information about the IP address. Accessing information like timezone and currency requires querying additional databases. This database also does not provide any information about VPN or proxy use, meaning the locations obtained for the given IPs could be wholly incorrect.
How to Geolocate an IP address with AbstractAPI
Before you geolocate a user's device via an IP address using Python, you should make sure it is a valid IP address. Python has several options for doing this, which we won't get into here.
Related: An in-depth look at validating IP addresses with Python.
Once you’re sure you have a valid IP address, you can use the AbstractAPI IP Geolocation API to turn that IP address into location information.
Getting Started With the API
The AbstractAPI Geolocation API is a REST API that accepts an IP address string and returns a response in JSON format, containing data about that IP address. It returns the device's location, carrier information, and other details.
Acquire an API Key
- To access the IP API, you'll need an API key. Go to the API documentation page, where you'll see an example of the API’s JSON response, and a blue “Get Started” button.
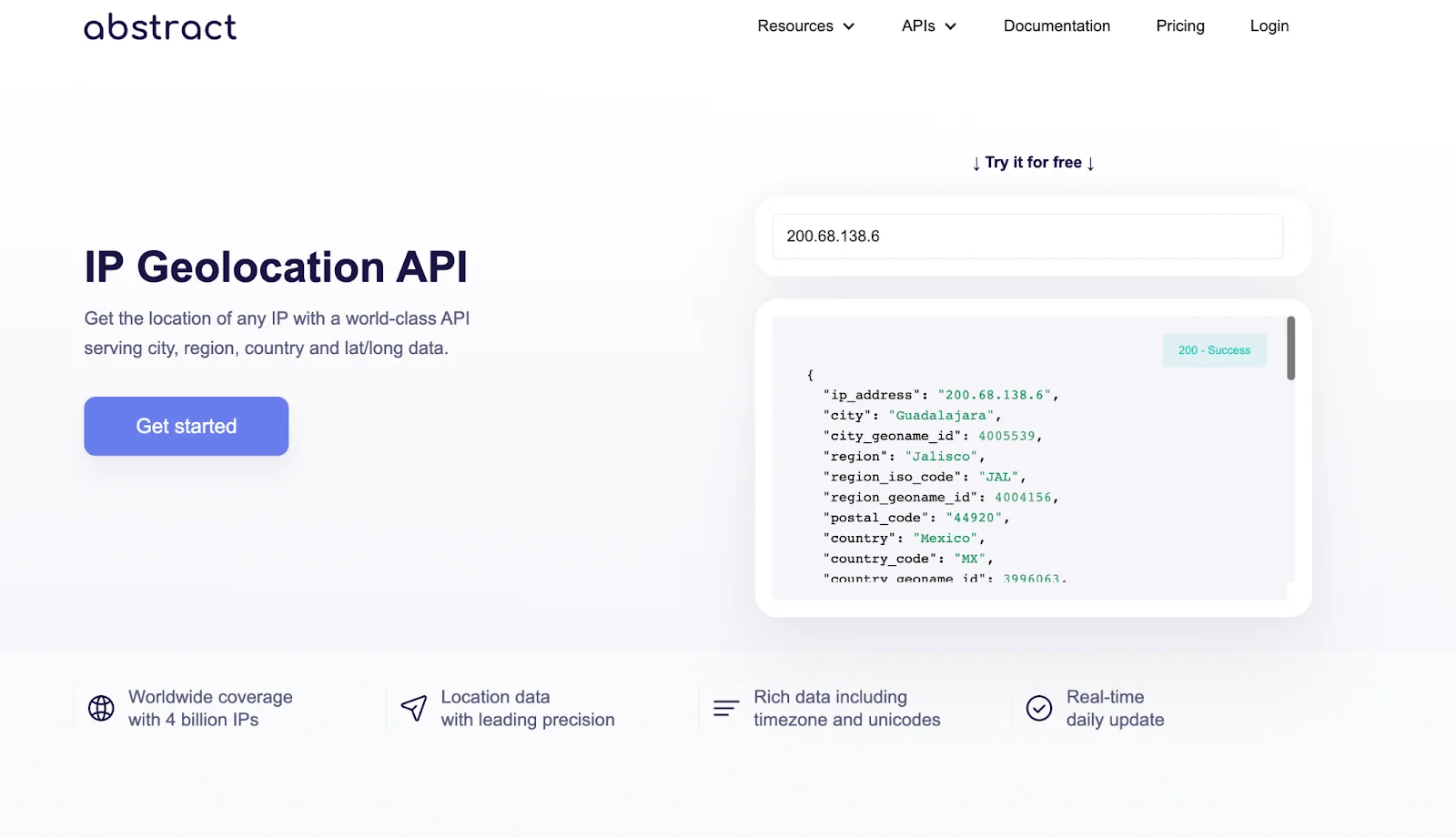
- Click “Get Started." If you haven't used AbstractAPI before, you’ll be asked to input your email and create a password.
- Once you’ve signed up or logged in, you’ll land on the API’s homepage. You'll see your API key, along with links to documentation, pricing, and support.
Sending an IP Geolocation Request
Making a request to the AbstractAPI endpoint is simple. All you need is your API key (also called an access token) and the endpoint's URL.
- Click "Try it Out" on the API dashboard. Then choose the “Python” tab on the "Try it Out" page.

- Create a function called get_geolocation_info in your Python request handler file and copy the code snippet into it.
We're using the Python requests library to send a GET request to the AbstractAPI URL. Add your token and the IP address as query string parameters and print the response that you get back from the API.
You can also include the token and IP address in a parameters object, and pass that object as the second argument to your requests library function as shown in the following code:
The response that AbstractAPI sends (which is printed when we call print(response.content)) looks like this:
In this example, we're just printing the response to the command line or console, but in a real application, you can pull the details you need (like the user's physical location, including the country name, city name, etc.) out of the object and use it in your Python application.
How IP Geolocation Works
"IP" stands for "Internet Protocol." Every device that is connected to the internet has a unique IP address. Because every IP belongs to a unique device, every IP address is unique. Every IP address contains hashed information about the device's current location.
How IP Addresses Wok
IP addresses come in two forms: IPv4 and IPv6. IPv4 addresses are four-part strings of numbers, separated by periods. IPv6 addresses are eight-part strings of numbers, separated by semicolons:
IPv4
IPv6
In both cases, IP addresses are assigned by your ISP (internet service provider) and uniquely identify your particular device to the network. Your IP changes depending on where you are, and what kinds of cables and methods are used to connect your device to the internet.
What is Geolocation?
Geolocation is the process of determining where a device is in the world via digital information acquired over the internet. This can mean either using the device's IP address or using GPS information about the device.

IP Geolocation vs GPS Geolocation
IP geolocation uses IP-to-location databases to determine which IP range the provided IP falls in. IP ranges are provided by ISPs. An IP geolocation db can return geographical information as accurately as to within what city the device is located.
GPS geolocation uses a combination of the device's internal GPS, cell phone towers, and WiFi connection points to triangulate a user's position. It is more accurate than IP geolocation, returning location information within a few feet of where the device is.
Geolocation Libraries for Python
Python libraries are modules that either ship as part of the Python core codebase, or can be added by downloading them via Pip, or from third-party sites like Github. A Python library typically tends to be well-maintained and documented, because there are so many people out there using Python.
Because of this, using a Python library for geolocation is a great idea. As mentioned, Python has several libraries to assist with translating IP addresses into location information. Let's take a look.
Best Python Libraries for Geolocation via IP
Here are five of the top libraries for translating an IP address using Python.
- GeoIP2 and MaxMind - the GeoIP2 module, downloadable via Pip, provides an SDK for the MaxMind online IP geolocation DB services.
- Ipregistry - the official Python client for the IPRegistry.io IP geolocation and threat intelligence API.
- Ipdata - this Python module validates and provides information about IP addresses through the ipdata.co geolocation and threat intelligence API.
- Flask - Flask is a Python framework for website development that includes tools for geolocation through IP addresses.
Other Geolocation Tools for Python
In addition to those tools, Python provides the following tools for working with IP addresses and geolocation data.
- GeoPy
- IP2Location
- Pygeoip
- GeoIP
Don't forget that before you do geolocation with an IP address, you should validate that IP address. You could build your own IP validation module using Regex in Python, however, this method is not recommended as it is error-prone and time-consuming. Instead, rely on an IP address validation tool (many of the tools listed above validate IP addresses.)
IP Geolocation APIs
API stands for "Application Programming Interface." An API is simply a set of rules and methods for integrating and communicating between two separate applications. These days, the term "API" has become synonymous with an online REST endpoint that returns data (such as geolocation data.)
Using online APIs for geolocation, as opposed to relying on built-in Python modules, can be a good way to keep package size down. You don't need to install any additional libraries or frameworks in order to interface with the API - all you need to do is send a GET request.
Best IP Geolocation APIs for Python
Here are some of the best IP Geolocation APIs:
- AbstractAPI Geolocation API - At AbstractAPI, we pride ourselves on being one of the top IP Geolocation API providers in the industry. Our IP Geolocation API is trusted by some of the world's largest companies, and we offer several paid tiers as well as a generous free option for testing and development purposes. Our paid tiers start at just $9.99/month for 20,000 requests, making it an affordable and reliable option for businesses of all sizes.
- Ipdata.co - One of the most accurate and reliable IP geolocator services available. Paid tiers start around $10/month, and the free tier offers enough bandwidth for testing the API.
- Maxmind - Is great for Python developers because there is a dedicated Python code module for Maxmind that can be downloaded via Pip. Also offers ad targeting and fraud protection services.
- IPRegistry.co - The best IP geolocator for threat intelligence needs. This API allows you to enrich online forms with IP data, target mobile users, detect VPN and proxy use, and perform Geo IP redirections.
- Ipgeolocation.io - A real-time IP locator that returns more detailed information than some of the other options on this list, including timezone, currency, sunrise/sunset times, flag, and more. Also allows for ISP lookup.
Using IP Geolocation for Web Development
Geolocation can be used for many things in website development. The most common use cases include determining a user's location for authentication purposes, displaying localized content, or showing a user their current location.
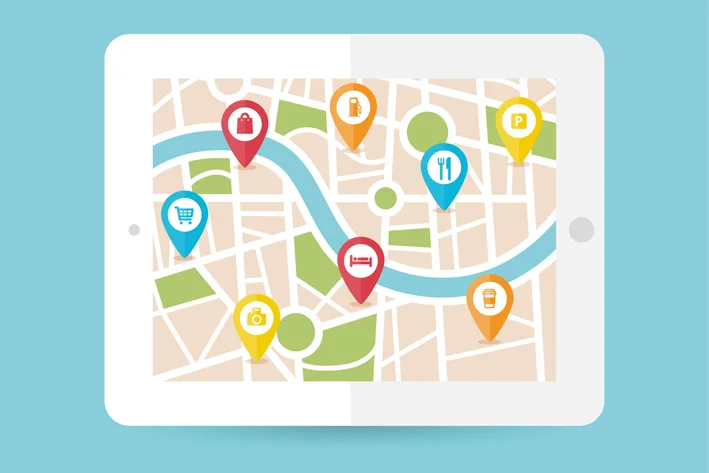
Geolocation-based web applications include mapping services like Google Maps and Waze, review, and business locating applications like Yelp, ride-sharing services like Uber, delivery services like Doordash, and many more. Any app, website, or marketer that needs to determine a user's location is likely using geolocation to do it.
But geolocation is also useful in many other ways. For example, a banking website might check a user's location when they attempt to log in and view their account. If the request is coming from an unexpected location, like another country, the bank can block the device from accessing sensitive information.
In addition, geolocation is used in geotargeting. Geotargeting is a way for advertisers to pinpoint the location of a user and show them the ads that are most relevant to them. Geotargeting can also be used to serve users content in their language, to show prices in their currency.
Best Practices for IP Geolocation
When doing IP address lookup with Python, it's important to adhere to some standards and best practices to protect your user's personal data.
- Ensure Data Privacy and Compliance - your website or application must follow data privacy and compliance laws, including GDPR laws. Your app or website must display a privacy policy outlining exactly what user data is collected, and how it is used.
- Accuracy and Precision of IP Geolocation Data - geolocation data obtained through IP addresses is not terribly accurate. You can only locate users within the limits of their city. It can't be used to look up a street address, phone number, user's name, sex, or any other identifying information.
- Updating and Maintaining IP Geolocation Data - if you store user location information, make sure that it is always up-to-date and accurate. It can be more trouble than it's worth to store location information, as users tend to move around a lot, and making geolocation IP requests is so easy to do that storing that data is usually unnecessary.
Limitations and Challenges of IP Geolocation
There are certain limitations to using an IP address to look up location information.
As previously mentioned, IP address locations are inherently inaccurate - a device can only be pinpointed to a city at best. IP location data provides 55-80% accuracy for a user's region or state and up to 75% accuracy for their city.
IP geolocation data comes with an accuracy radius. The actual geolocation of an IP address is within this radius.

IP geolocation services tend to work better in big cities than in smaller ones and rural areas.
Finally, if users are using VPNs or other types of services that mask their actual IP addresses, IP geolocation becomes useless.
Conclusion
IP geolocation is incredibly useful for many things in website and application development. It can be used to show users maps, localized content and ads, as well as perform authentication checks.
Every device with an internet connection has a unique string of digits that identifies it to the network. IP locations change depending on where a device accesses the internet. These unique strings can be used to geolocate a user's device fairly accurately but not exactly.
Python, which is one of the most prevalent coding languages for web development, has many tools and libraries available for going geolocation with IP addresses. When using Python for geolocation, it's important to adhere to best practices and standards to protect user data.
FAQs
What is IP Geolocation?
Geolocation is the process of determining a device's physical location based on digital information found on the internet. This can involve looking up the device's IP address and translating that address into location data. An IP address is a unique string of numbers that identifies a particular device to the network.
What are the best Python Libraries for IP Geolocation?
Some of the best python library options for doing IP geolocation include GeoPy, IP2Location, Pygeoip, GeoIP, MaxMing, and IPRegistry.
What are the best IP Geolocation APIs for Python?
Some of the best IP Geolocation APIs for Python include AbstractAPI's Ip Geolocation API, Radar's Geocoding API, Foursquare's Location Data API, and IPStack.
How can IP Geolocation be used in cybersecurity?
Geolocation can be used to pinpoint a user's location to determine if a request is coming from an expected client or from a potential bad actor. It can also be used to determine if a user is using a proxy, VPN, or other IP-obscuring technology, and can identify fraudulent activity.
How can Geolocation be used for business intelligence?
Geolocation can be used to determine user behavior based on their region and target efforts toward areas where those efforts will see the greatest payout. For example, if users in Thailand frequently purchase one type of product vs users in China, you can push more ads for that product to Thailand to increase revenue.