Setting up CakePHP
CakePHP is a PHP web framework that follows the popular MVC (Model View Controller) architecture. Initially written in 2005, it has enjoyed a good following since then and has amassed over 8k stars on GitHub. CakePHP is under active development and is used by many small and large enterprises.
Installing CakePHP
CakePHP can be installed as a dependency for a PHP project by running the composer, the de-facto PHP dependency manager. Let’s create a new PHP project with CakePHP. Before starting, make sure to have one of the latest versions of :
- PHP (version 8.1 or later).
- Composer (version 2.3 or later).
Open a command line terminal and change to a suitable directory. After that, run the following composer command to create a new PHP project config with the CakePHP framework.
This command will create a new project named ‘CakeEmailValidator’ and install the latest CakePHP version, among other dependencies. A top-level directory with the same name is created in the current path. The sub-directory structure of this directory is as follows.

Testing CakePHP
This project contains a skeletal CakePHP web app you can test using the built-in development server. Run the following command to start the server.
This command will start the web app on port 8765 with a valid URL. Point your browser to the URL http://localhost:8765/, and you should see the default web page of the app.

Understanding Validations in CakePHP
CakePHP provides validation classes with a rich set of off-the-shelf validation rules for validating input data submitted through web forms. In the case of a form containing an email field, you have multiple approaches to validate it within CakePHP.
- Default validation: Using the Validation::email( ) method to validate the email format, with an option to perform deep validation for checking the email domain and MX records.
- Custom Validation: Using the class Validator to define custom rules related to email id format, domain, or additional regex based rules.
- API Validation: Using the class Client to make an API call to an external email validation service.
Let’s build a demo email validation application using all three options.
How to Validate Email Field in CakePHP
There are always two parts to a web application: the front end and the back end. Let's first build the frontend web page for validating the email field. In a CakePHP project, the frontend views are stored in the 'templates' subdirectory within the project directory.
Inside the 'templates' subdirectory, create a new directory named 'emailValidator' and create a new file named 'index.php' within that directory. Open this file in a code editor and replace the content with the below code.
File: ‘templates/emailValidator/index.php’
This code adds a simple HTML form with an email field and a submit button. A separate <div> section is added to conditionally display the list of errors based on the response from the backend after form submission.
This front end will be used for all the validation demonstrations you will see later in this article.
Now, to handle the form submission at the backend, run the following command from another terminal after changing the directory to the top-level ‘CakeEmailValidator’ directory.
This command creates a new controller within the CakePHP project under ‘src/Controller’ path. This is a PHP source file.

Open the new PHP controller file in a code editor and replace the default code with the following code:
File: ‘src/Controller/EmailValidatorController.php’
This code adds a new method of action named index, which gets executed upon form submission from the front end. This code first checks if the request method is a POST request. It ensures the code inside the if block is executed when the user submits the form. After that, it retrieves the user-provided email from the form data submitted via POST.
For validating the email field, this code uses the first approach of default validation presented earlier. It imports the built-in CakePHP Validation class and calls the email( ) method, which returns a boolean value.
Upon saving this file, your CakePHP application has a new URL path, ‘http://localhost:8765/emailValidator/index’ for performing default validation on email.
Open this URL in the browser and test it by submitting an email address. You should see success and failure responses for valid and invalid email address formats, respectively.

Advanced Email Validation Techniques
The default validation is good when you want to guard against junk input data that does not resemble an email address. But if you enforce specific rules for creating or accepting email addresses, you need CakePHP's custom validation features.
To create a custom validation rule, define a new validation method in the EmailValidator Controller and name it customValidation(). Add this method within the EmailValidatorController class with the following logic.
File: ‘src/Controller/EmailValidatorController.php’
Note: As a good coding practice, all validation logic should be defined in separate models and controllers. However, for the sake of simplicity, the code presented here is contained in a single controller.
The customValidation() method creates a Validator object that defines two custom rules in addition to the default validation.
- allowedDomains: This rule enforces that the email address should contain only a list of allowed domains. In this code, the rule only allows “gmail.com” and “outlook.com” domains. In this way, you can restrict the submission of email addresses with specific domain names only.
- noMultipleDash: This rule enforces that the email address should not contain any dash , ‘-’ symbols. This rule is helpful in case you want to restrict email addresses having special characters.
When this code is triggered, the Validator object runs through these custom rules in sequence to check the email syntax and accumulates the errors in the $errors variable based on the bool return value of each rule. This data is passed on to the front end for displaying on the web page.
You can also add more custom rules using the add( ) method of class Validator for alphanumeric, numeric, regular expressions, and other validation checks.
Before saving this file, add this line of code to import the class Validator, just below the namespace declaration at the top.
This new validation method is now accessible from the URL http://localhost:8765/emailValidator/customValidation. Open this URL on the browser, and now you can test these rules.

A Better Solution: AbstractAPI
Having custom rules for email address validation is a good idea, but sometimes, it's a better approach to rely on a reliable API service. The Abstract Email Validation & Verification API is one such reliable and robust service that offers a host of options to verify and flag emails based on several criteria.
You can sign up for the Abstract account and get access to 100 free requests by using the API key that is assigned to your account upon logging in to the API dashboard page.
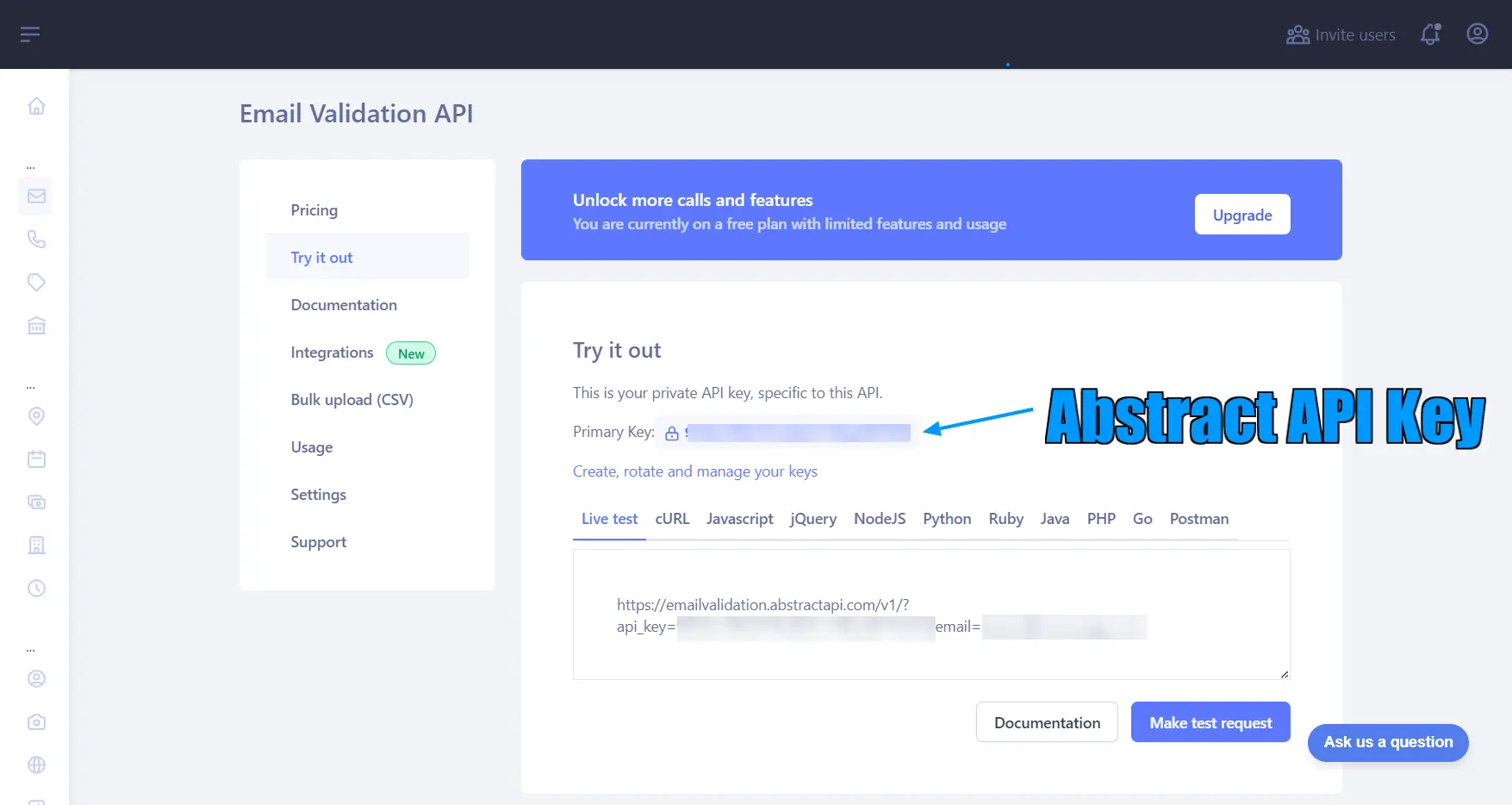
Let’s integrate the Abstract Email Validation and Verification API. To achieve that, define one more method named abstractAPIValidation( ).
File: ‘src/Controller/EmailValidatorController.php’
This method uses the CakePHP’s Client class from Http namespace. Make sure to include it at the top after the namespace declaration.
This method makes an HTTP GET request to the Abstract API endpoint and retrieves the response to parse the validation status. In this code, the validation is limited to checking the email address format and SMTP to ascertain the email address domain’s mail server capabilities. But you can also add additional validations as per the documentation of this API.
Before saving the file, replace the placeholder <ABSTRACT_API_KEY> with your Abstract account’s API key. Now you have one more URL endpoint to test this validation: http://localhost:8765/emailValidator/abstractApiValidation.
Here is how you can test it for invalid and valid email addresses.
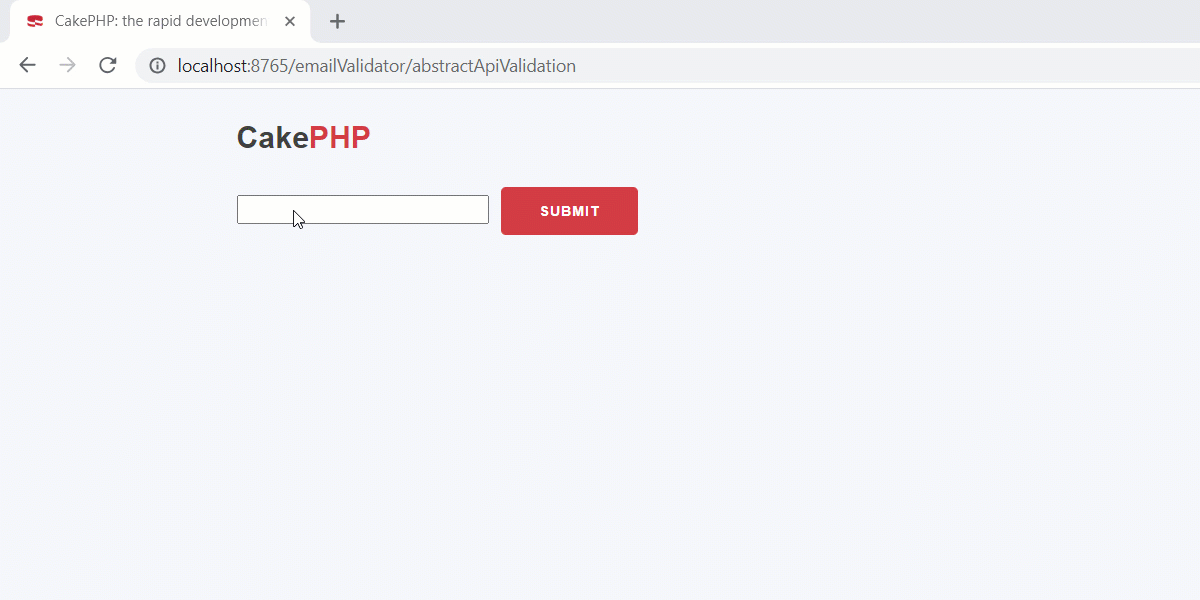
Here is the complete EmailValidatorController.php file with the three email validation options you have just learned.
Conclusion
We have shown you how to validate email addresses within a CakePHP application. You can choose one of the three options depending on your use case. While the default and custom validation are suited for general validations, they do not guarantee the deliverability and quality of email addresses. With Abstract Email Validation and Verification API, you have these options, which would otherwise require you to send a test email.
FAQ
How to validate email using CakePHP?
CakePHP offers a few validation options specifically for email addresses. For basic validation, you can use the email( ) method from the Validation class. It can perform basic email address format checks. For custom validation involving filtering of email addresses based on domain names and special characters, you can use the Validator class. Using the add( ) method of this class, you can include custom validation rules related to email address length, characters, and domains. It gives you complete control over the input data submitted through web forms, be it email addresses or any other form of data. For more legitimate validation, you can use the Abstract Email Validation and Verification API, which lets you ascertain the email address's deliverability without sending a test mail.
How do you verify and validate email addresses in PHP?
PHP offers some built-in functions for data validation. In the case of email, you can use the filter_var( ) function. It accepts several pre-configured filter types, including FILTER_VALIDATE_EMAIL, for validating email addresses. If you are building a PHP application using some popular frameworks, you have more options. For example, in the case of CakePHP, you can use CakePHP's Validator class to define custom rules. Alternatively, you can also use an email validation API. The Abstract Email Validation and Verification API offers a robust, reliable, and authentic service for validating email addresses. It provides much information about any email's deliverability, domain, quality, and other traits.
What are the email validation rules in CakePHP?
Under CakePHP's Validation namespace, there are a few classes that are designed to offer different ways of validating email addresses. The Validation class contains methods for validating pre-formatted data like email address, URL, date, hex color code, etc. For email, you can call the email( ) method to perform basic format validation of an email address. For more granular validation, down to the email id and domain level, you can leverage the Validator class with custom rules. You can also build more complex validation logic using the ValidationRule and ValidationSet classes.