Understanding Email Validation
Email validation is the process of determining whether a given email address is correct, valid, and deliverable. That means everything from making sure there are no typos in the address, to checking MX records for the domain, to making sure the address isn’t a temporary address.
Angular provides some nice out-of-the-box validation solutions using form control and HTML5 input validation that you can easily implement in your app’s frontend to check for typos and malformed strings.
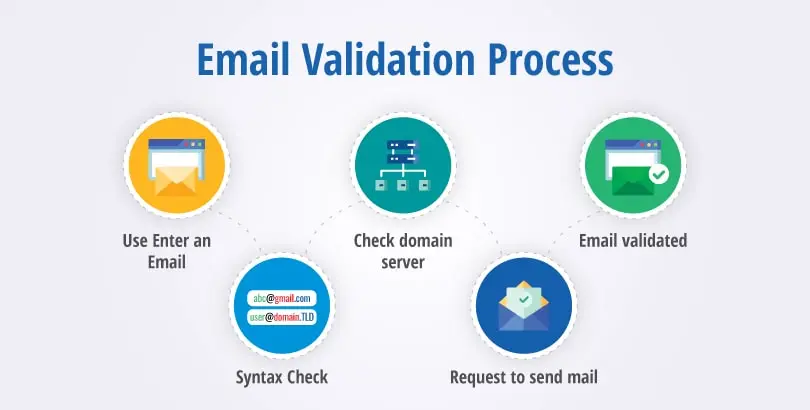
Implementing Angular Email Validation
How does Angular or AngularJS validate email address strings? There are three ways to validate email addresses using Angular:
- EmailValidator - Angular’s built-in validation tool that checks whether a given string is an email address
- PatternValidator - Angular’s validation tool that allows you to provide a RegEx pattern to use as a validator
- CustomValidator - your own custom-built email validator built using ng-model.
Before we look at how to implement each method, let’s touch on template-driven forms vs reactive forms.
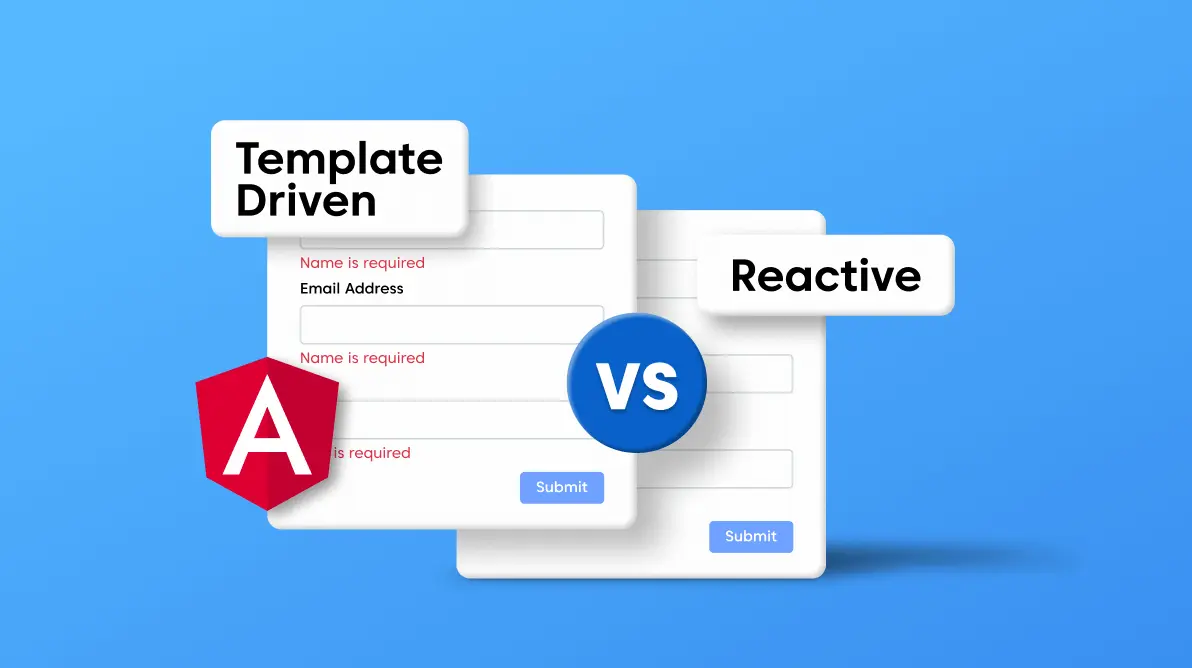
Template Driven Forms
Template-driven forms are very similar to what AngularJS provides. A template-driven form uses the two-way data binding of ng-model to sync an HTML form with a data model.
To use template driven forms in Angular, you must import the directive into your root App file:
Now you can build template-driven forms in your application like so:
The logic for this form (i.e. what happens when the form is interacted with and submitted) is put into an attached component class:
Here, you can see that all we’re doing on submit is logging the data from the form. In a real app, this would be where you send your data to the server.
Reactive Forms
Reactive forms are implemented in a very similar way to template-driven forms, but you must import a different module for them:
Now, you can build a reactive form using the following format:
As you can see, the difference is that the entire form is bound to a single variable in the data model via the formGroup directive and given a form name. Also, the required attribute has been left off the input - this is because validation will now happen inside the component class:
Here, we see the validators being applied to the email field of the form variable via a FormControl and we see that the submission of a form is handled by submitting the entire form at once.
For the purposes of this tutorial, we’ll use template-driven forms. For a deep dive into template-driven vs. reactive forms, check out this blog post.
EmailValidator
You can use the EmailValidator module in a template form without even having to handle validation in the component class. All the validation is handled by the form itself:
Because we’ve applied the email attribute to the input, Angular will handle all validation of the address to make sure the string matches what Angular expects to be a valid email address and update the email model accordingly.
In the div we can examine the model and show an error message to the user if the model’s error.required or error.email field contains any required or email errors. We've added a class of error to the div so we can use CSS to style the error message appropriately.
The required attribute is similar to AngularJS’s ng-required directive. You could also add minLength and maxLength attributes to validate the length of the input.
Angular will even handle disabling the Submit button for us if the form is invalid (similar to using ng-disabled in AngularJS.) In this example, all that would need to be included in the component class is the mySubmit logic to handle submission.
PatternValidator
Unfortunately, Angular’s email attribute is not 100% reliable at detecting invalid email addresses. For example, it accepts abc.abc as a valid email address.
For a slightly more reliable method, we can use RegEx to do pattern validation (see later paragraphs for why this is still not a great solution.) This is similar to AngularJS’s ng-pattern directive.
Instead of using the email attribute on the input, we’ll instead add a pattern attribute and provide our own custom RegEx string to compare to the email string for validation:
Angular will now evaluate anything that is typed into the input against the RegEx pattern you provided, and use that to determine whether or not the email is valid.
Custom Validator
A much more robust method for doing email validation is to create your own custom validator in the component class and use that for validation. This way, you can use an external validation API or your own validation code to validate the addresses (we’ll look at how to use AbstractAPI to validate email addresses in a later section.)
To create a custom validator, define it in a separate directive file. In this example, we’ll name our file email-validator.directive.ts.
This factory accepts a regular expression as an argument and returns a function that uses that regular expression to test the email field of a passed Form Control. It’s a reusable piece of code that can be applied to any email input textbox in your application.
AngularJS Email Validation
The primary difference between Angular and AngularJS is that Angular is based on Typescript while AngularJS is based on Javascript. Typescript is just a more strongly opinionated form of Javascript that allows you to use static typing and interfaces. Typescript is also a little more readable and more easily maintained than Javascript.
AngularJS email validation is very similar to Angular template-driven validation. There are just a few differences in the names of modules. Here is an example of using the EmailValidator module in AngularJS:
Validation Patterns and RegEx
The problem with using RegEx to validate email addresses is that it is impossible to come up with a regular expression that captures all possible valid or invalid email addresses. These days, especially with unique top-level domains being used everywhere, it becomes very difficult to predict what a valid email address looks like.
For example, the following are all valid email addresses:
user@tyes.media
user@met.museum
user@gmail.com
user@hype.io
In order to allow all those addresses to pass as valid, you would have to write an insanely long RegEx pattern, and you still probably won’t get it right.
The other issue with using RegEx for email validation is that it doesn’t check things like MX records, SMTP, deliverability scores, or whether the email is a role-based email, catch-all email, or temporary address.
A Better Solution: AbstractAPI
Using an email validation API is the only way to ensure not only that the string the user provided you is free from typos or errors, but that the address is valid, deliverable, and active. Let’s quickly walk through how to implement AbstractAPI in your Angular custom validator.
Get an API Key
To use the API, you’ll need an API key. You can sign up for a free account with Abstract using an email and password. Navigate to the Email validation homepage and click ‘Get Started.’

From there, you’ll be taken to the API dashboard where you’ll see your API key and links to documentation, customer support, and pricing.
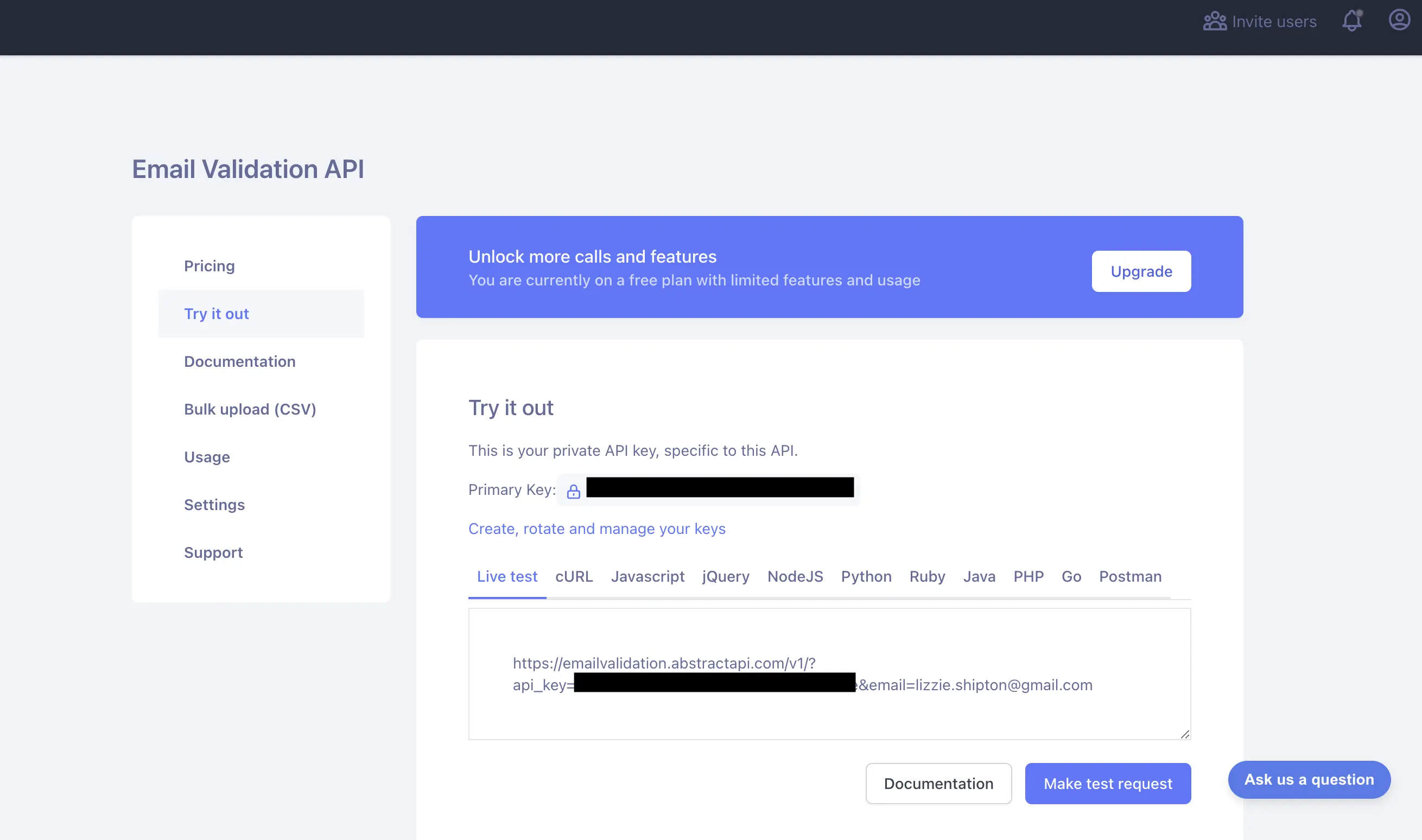
Copy the API key. You’ll need to use it in your validator.
Create an Async Validator
Using an API to validate your email addresses means you’ll be making a network request, so you’ll need to write a validator that implements the AsyncValidator interface.
First, make the HttpClient module available in your app by importing it into the AppModule and adding it to the imports array:
and
Next, create a custom async validator that uses the HttpClient to send a GET request to the AbstractAPI endpoint. You’ll pass the email for validation and your API key as query string parameters.
The API will return a JSON response object with data about the email and a boolean field telling you whether it is valid or not.
In our validation example, we only looked at the is_valid_format field, but you should examine each of the relevant fields to determine whether or not the provided email is valid.
Common Issues and Solutions
The primary concerns when implementing email validation into your forms is to make sure you are handling errors correctly, displaying relevant error messages to the user, and disabling inputs and buttons if the user has not input a valid address.
Angular can handle a lot of this for you through the errors and disabled fields, but it is your job to make sure the logic is correct for when things are disabled, and the messages you are displaying to users make sense.
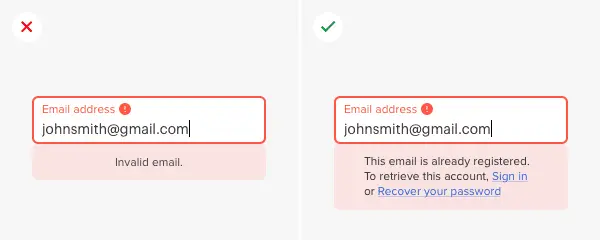
Another common issue developers run into is using RegEx patterns that don’t catch all invalid emails, or don’t allow valid emails to pass. This is why you should never rely solely on RegEx for your email validation. RegEx is fine to use as a typo checker, but that should only be one line of defense in your filtering of invalid addresses.
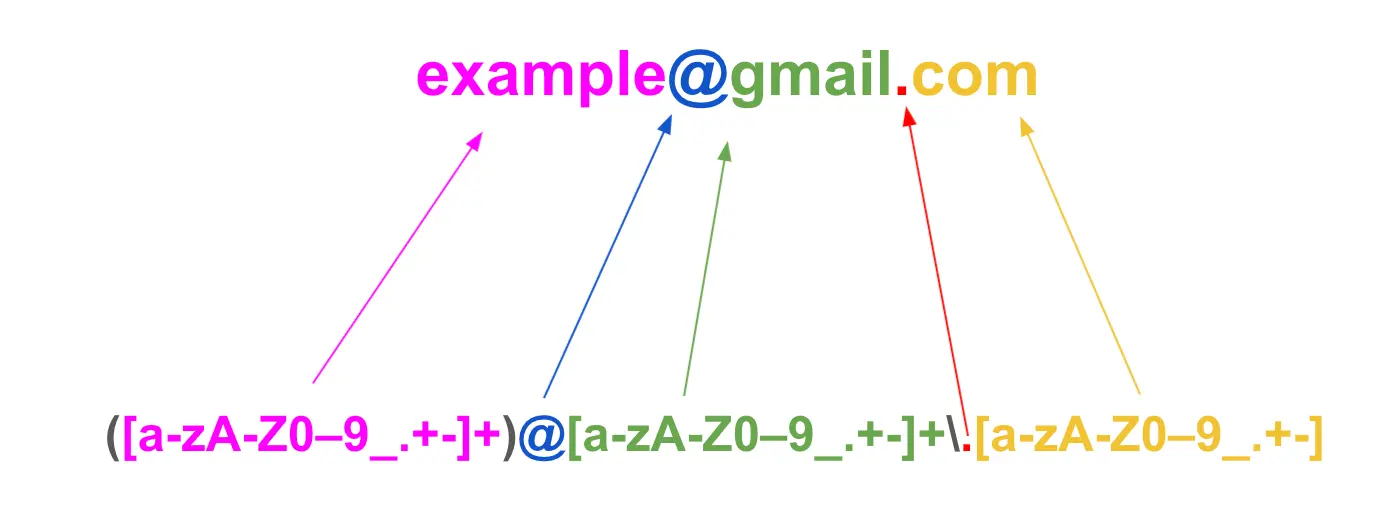
Conclusion
Angular makes email validation quick and simple. When used in combination with an email validation service like AbstractAPI’s Free Email Validation API, it is a powerful way to prevent invalid emails from clogging up your precious email list.
FAQs
How do you validate an email address in Angular?
There are three ways to validate an email address in Angular: using the built-in EmailValidator module, using the built-in PatternValidator module and providing a RegEx pattern, or using the CustomValidator module and writing your own validation function using an API or other email validation service.
What is the difference between Angular and AngularJS in terms of email validation?
The primary difference between Angular and AngularJS is that Angular uses Typescript while AngularJS uses Javascript. That means everything in your Angular app must be strongly typed, and you have access to interfaces and other helpful Typescript things.
Angular also introduced the concept of ‘reactive forms’ which means you can bind your entire form to a data model and pass custom validators directly to the component class for custom validation.
Can you use regex for email validation in Angular?
You can. The question is ‘should you’? And the answer to that is, no. At least, not only RegEx. RegEx is fine for evaluating a string for typos, but it should not be relied upon to perform email validation, as it does not tell you whether MX records are valid, or whether the email address is a catch-all, role-based, or temporary email. Additionally, with the prevalence of custom top-level domains, it would be impossible to write a regular expression that correctly allowed all valid email addresses through.