What is API Development?
API (Application Programming Interface) development refers to the process of designing, building, and maintaining APIs that enable different applications, services, and systems to communicate and exchange data seamlessly. APIs act as intermediaries, allowing developers to integrate functionalities without needing direct access to the source code of an application.
For example, when you use a mobile app to check the weather, it retrieves real-time data from an API rather than storing it locally. Similarly, payment gateways like PayPal or Stripe use APIs to enable secure transactions between e-commerce websites and banking systems.
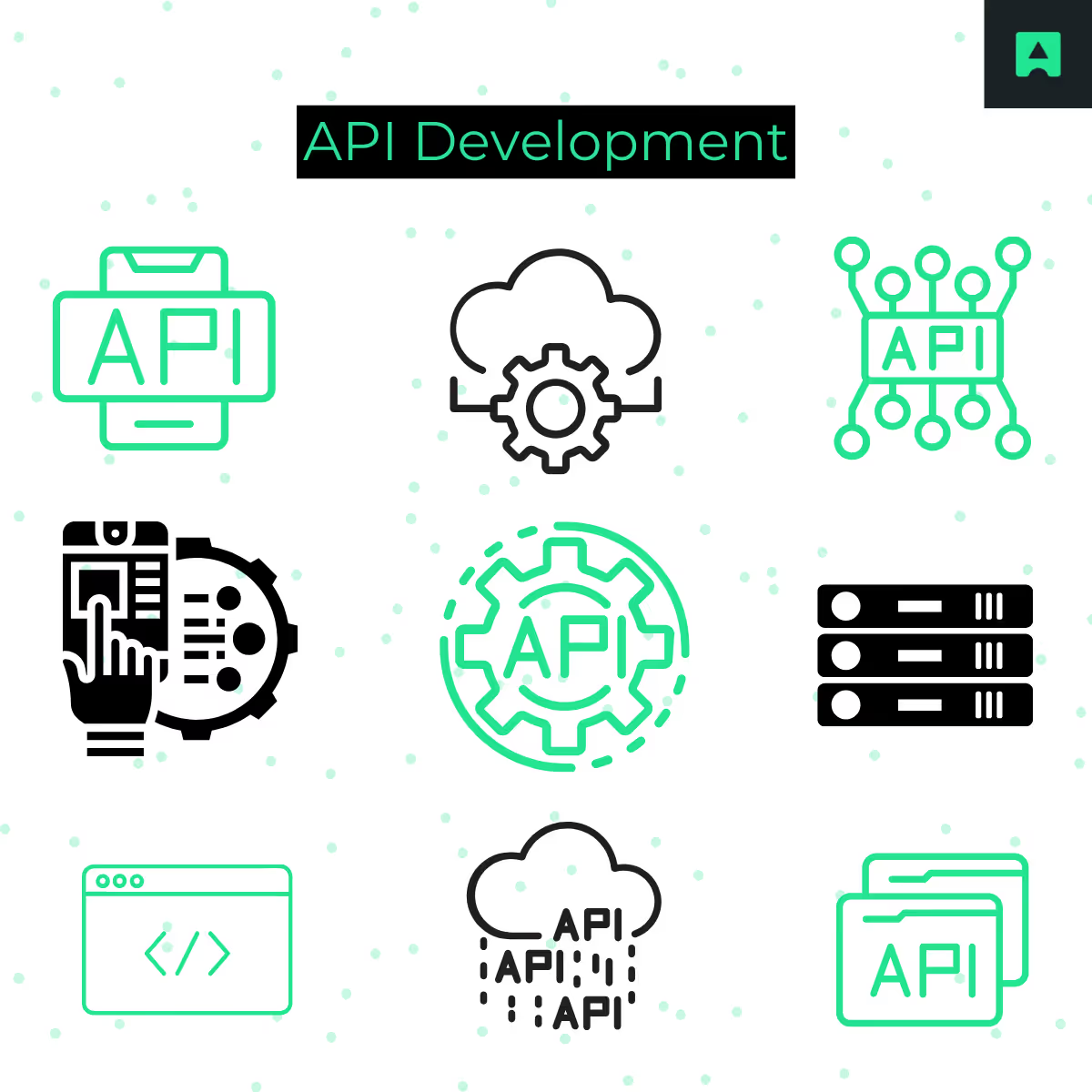
What is the Importance of APIs in Modern Software Architecture?
APIs play a critical role in today's digital landscape, supporting automation, integration, and scalability. They serve as the backbone for web applications, mobile apps, IoT devices, and cloud-based solutions.
The key benefits of APIs include:
- Automation: APIs enable seamless interactions between software applications, reducing the need for manual input.
- Integration: Businesses can integrate third-party services (e.g., payment gateways, social media, CRM tools) to boost the user experience.
- Scalability: APIs help companies scale their services by enabling modular development and microservices architecture.
- Innovation: Open APIs allow developers to create new applications and services, fostering technological advancements.
Key Components of APIs
Endpoints of Key Components of APIs
API endpoints are specific URLs where applications send requests and receive responses. They define the point of interaction between the client and the API. Each endpoint is designed to handle specific data transactions, ensuring structured communication between applications.
For example, a weather API may have an endpoint like:
GET https://api.weather.com/v3/weather/forecast
HTTP Methods (Verbs) of Key Components of APIs
APIs use different HTTP methods to define the type of operation performed:
- GET: Retrieves data from the server.
- POST: Sends new data to the server.
- PUT: Updates existing data.
- DELETE: Removes data.
- PATCH: Partially updates data.
Requests & Responses of Key Components of APIs
APIs process client requests and return structured responses in formats like JSON or XML.
A sample request for retrieving user details may look like this:
GET /users/123 HTTP/1.1
Host: api.example.com
Authorization: Bearer token123
The server responds with structured data:
{
"id": 123,
"name": "John Doe",
"email": "johndoe@example.com"
}
Authentication & Authorization of Key Components of APIs
API security is essential to prevent unauthorized access and data breaches. Implementing robust authentication and authorization mechanisms is crucial for protecting APIs from security threats such as unauthorized data access and credential leaks.
Authentication verifies the identity of a user or application, while authorization determines what actions they can perform.
Common security mechanisms include:
- API Keys: Unique identifiers assigned to clients for accessing an API. These are simple but can be vulnerable if exposed.
- OAuth 2.0: A secure authorization framework that allows third-party applications to access user data without exposing credentials. OAuth enables the use of access tokens, reducing the need for password sharing.
- JWT (JSON Web Tokens): Encoded tokens used to authenticate and authorize users. These tokens contain user information and expiration details, making them efficient for stateless authentication.
- HMAC (Hash-based Message Authentication Code): A technique that ensures data integrity by generating a cryptographic hash of the request payload, making it tamper-proof.
Rate Limiting & Throttling of Key Components of APIs
To prevent API abuse and ensure system stability, developers implement these techniques, APIs maintain optimal performance, prevent downtime, and reduce the risk of denial-of-service (DoS) attacks.
Let’s check the techniques:
- Rate Limiting: Restricts the number of API requests a client can make within a specified time frame (e.g., 100 requests per minute).
- Throttling: Slows down excessive API requests instead of outright blocking them, ensuring fair usage without overwhelming the server.
- Quota Management: Assigns specific API request quotas to users or applications based on their subscription plans or usage patterns.
API Development Process
1. Planning
API development begins with a clear plan that outlines the purpose of the API, its target audience, and business objectives.
This phase includes:
- Identifying stakeholders and their requirements to ensure the API meets business and user needs.
- Defining core functionalities and expected use cases to guide design decisions.
- Establishing security policies to prevent unauthorized access and protect sensitive data.
- Setting performance expectations to handle traffic loads efficiently.
- Ensuring compliance with industry regulations such as GDPR, HIPAA, or PCI-DSS to protect user data.
- Creating a roadmap for development milestones, version releases, and long-term maintenance.
2. Design
Designing an API is a crucial step that determines its usability, performance, and security.
Considerations in this phase include:
1. Choosing an API architecture:
- REST: A stateless architecture that uses standard HTTP methods and is widely adopted.
- SOAP: A protocol-based approach with strict specifications, suitable for enterprise applications.
- GraphQL: A flexible query language that allows clients to request specific data.
2. Defining endpoints, request parameters, and response structures to ensure consistency and clarity.
3. Selecting authentication mechanisms such as OAuth, JWT, or API keys for secure access.
4. Ensuring proper error handling with descriptive error messages and appropriate HTTP status codes.
5. Prioritizing documentation to assist developers in integrating the API easily.
6. Optimizing data formats by choosing between JSON and XML based on efficiency and readability.
3. Implementation
Once the design is finalized, the actual development process begins. Key steps include:
a) Selecting the right programming language and framework based on the use case:
- Node.js with Express for fast, lightweight APIs.
- Python with Flask or Django for robust web APIs.
- Java with Spring Boot for enterprise-level applications.
b) Building API logic to handle incoming requests, process data, and return structured responses.
c) Implementing security measures such as input validation, encryption, and role-based access controls.
d) Optimizing performance with caching strategies, database indexing, and minimized payload sizes.
e) Ensuring scalability by designing for horizontal scaling and load balancing.
4. Testing
Comprehensive testing ensures API reliability. Essential testing methods include:
- Unit testing to validate individual API functions.
- Integration testing to check compatibility with other services.
- Load testing to simulate high traffic scenarios and evaluate performance.
- Security testing to identify vulnerabilities such as SQL injection and unauthorized access.
- Automated testing using tools like Postman, JUnit, and SoapUI for continuous quality assurance.
5. Deployment & Maintenance
API deployment and maintenance involve:
- Hosting options: Cloud-based, on-premise, or hybrid solutions.
- Setting up API gateways for traffic management and security.
- Implementing monitoring tools (e.g., Prometheus, Datadog) to track performance.
- Regular security updates to patch vulnerabilities and comply with regulations.
- Versioning strategies to ensure backward compatibility and smooth transitions.
Take note!
By following a structured development process, APIs can be scalable, secure, and easy to maintain.
Understanding Different Types of APIs
APIs (Application Programming Interfaces) enable seamless communication between different software applications. They play a crucial role in modern digital ecosystems, providing connectivity, automation, and scalability. Here, we explore the four main types of APIs and their applications.
Open APIs (Public APIs)
Open APIs, also known as public APIs, are accessible to external developers and organizations. These APIs encourage innovation by allowing third-party developers to integrate with services and build new applications.
Key characteristics of Open APIs include:
- Easy accessibility: Available for external use with minimal restrictions.
- Standardized documentation: Helps developers understand implementation.
- Encourages third-party integrations: Expands the functionality of existing platforms.
Take note of these examples: Google Maps API, Twitter API, and Stripe API.
Internal APIs (Private APIs)
Internal APIs are designed for use within an organization. They facilitate internal software integrations and improve efficiency by enabling secure data sharing between teams and systems.
- Restricted access: Only available to internal teams.
- Enhanced security: Not exposed to external developers.
- Optimized for internal workflows: Streamlines internal operations and data handling.
Take note of these examples: A company’s HR system API that integrates with payroll software.
Partner APIs
Partner APIs are shared with specific business partners, allowing controlled access to certain functionalities. These APIs enhance collaboration and business-to-business integrations.
- Restricted but external access: Only available to trusted partners.
- Secure authentication: Uses API keys, OAuth, or other authentication mechanisms.
- Facilitates business collaboration: Enables seamless integration between partner services.
Take note of these examples: Payment gateway APIs used by e-commerce platforms.
Composite APIs
Composite APIs allow multiple API calls to be combined into a single request. This improves efficiency and performance by reducing the number of requests sent to the server.
- Increase efficiency: Reduces load time and improves responsiveness.
- Data aggregation: Retrieves data from multiple endpoints in one call.
- Ideal for microservices architecture: Simplifies interactions between different services.
Take note of these examples: A travel booking API fetching flight, hotel, and car rental details in one request.
Features of Efficient APIs
Not all APIs are created equal. High-quality APIs must meet specific efficiency standards to ensure smooth operation and developer satisfaction. Below are the essential features of an efficient API.
1. Scalability
A well-designed API should be able to handle increasing user demand and growing data volumes without performance degradation.
- Load balancing: Distributes traffic efficiently across servers.
- Rate limiting: Controls API usage to prevent overload.
- Cloud-based architecture: Allows flexible resource allocation.
2. Security
Security is critical to protect sensitive data and ensure authorized access.
- Authentication mechanisms: OAuth, API keys, and JWT for secure access.
- Data encryption: Protects information in transit and at rest.
- Access control: Role-based permissions to restrict sensitive operations.
3. Consistency
A consistent API structure ensures a smooth developer experience and minimizes errors.
- Standardized naming conventions: Ensures uniformity across endpoints.
- Error handling: Returns meaningful and structured error messages.
- Version control: Maintains compatibility with older API versions.
4. Performance Optimization
Optimized performance leads to faster response times and a better user experience.
- Caching strategies: Reduces redundant API calls.
- Minimized payload sizes: Uses lightweight data formats like JSON.
- Efficient database queries: Improves data retrieval speed.
5. Clear Documentation
Comprehensive and well-structured documentation is essential for API adoption.
- Interactive guides: Includes examples and code snippets.
- API testing tools: Allows developers to test endpoints easily.
- Consistent updates: Keeps documentation aligned with API changes.
Conclusion of Efficient APIs
APIs are the backbone of digital transformation, enabling seamless integration across platforms and applications. Understanding the different types of APIs—Open, Internal, Partner, and Composite—helps organizations choose the right solutions for their needs. Additionally, ensuring APIs are scalable, secure, consistent, high-performing, and well-documented improves their effectiveness and usability.
By prioritizing these features, businesses can create powerful APIs that drive innovation and efficiency.
API Deployment and Maintenance: Best Practices for Reliability and Security
APIs (Application Programming Interfaces) play a crucial role in modern software development, enabling seamless integration between applications. However, deploying and maintaining an API requires careful planning to ensure security, scalability, and performance. In this guide, we’ll explore key deployment considerations, maintenance strategies, and testing practices to keep your API running efficiently.
Deployment Considerations
When deploying an API, choosing the right hosting environment and implementing an effective traffic management strategy are essential.
What are the Hosting Options?
Selecting the appropriate hosting model depends on your project’s requirements, budget, and scalability needs. The three primary hosting options are:
- Cloud-Based Hosting: Services like AWS, Google Cloud, and Azure provide scalable, flexible solutions with managed infrastructure, reducing operational overhead.
- On-Premise Hosting: Ideal for organizations with strict security requirements, this option provides complete control over API deployment but requires in-house infrastructure management.
- Hybrid Solutions: A combination of cloud and on-premise hosting, hybrid setups offer the flexibility of cloud computing while maintaining on-premise security controls.
API Gateway Setup
An API gateway acts as a centralized entry point for API requests, handling:
- Traffic Management: Distributes requests efficiently to prevent overload.
- Security: Implements authentication, authorization, and threat protection measures.
- Rate Limiting: Controls request volumes to prevent abuse and ensure fair usage.
- Logging and Monitoring: Tracks API activity to identify potential issues and optimize performance.
Maintenance Strategies
Proper maintenance ensures the long-term stability and security of an API. Here are key strategies for effective API management:
Monitoring and Analytics
Continuous monitoring helps track API performance and detect issues before they escalate. Key aspects include:
- Uptime Monitoring: Tools like Pingdom and New Relic ensure your API remains accessible.
- Performance Metrics: Track response times, error rates, and request volumes.
- User Analytics: Understand API usage patterns to optimize performance and scaling.
API Versioning
To maintain backward compatibility and avoid breaking changes, consider these versioning approaches:
- URL Versioning: Example: /v1/resource and /v2/resource.
- Header Versioning: Clients specify the API version via HTTP headers.
- Query Parameter Versioning: Example: ?version=1.0 in the request URL.
Security Updates and Compliance
Regular updates and adherence to security best practices protect against vulnerabilities.
Focus on:
- Encryption: Use TLS/SSL to secure data in transit.
- Authentication & Authorization: Implement OAuth, API keys, and JWT tokens.
- Compliance: Ensure adherence to GDPR, HIPAA, and other relevant regulations.
API Testing Best Practices
Testing is crucial to ensuring API reliability, security, and performance.
Different testing methodologies address various aspects of API functionality.
Functional Testing
Ensures API endpoints return expected responses based on input parameters. This includes verifying request and response formats, status codes, and data accuracy.
Load Testing
Evaluates API performance under different levels of traffic to determine scalability limits. Popular tools include JMeter and Gatling.
Security Testing
Identifies vulnerabilities such as:
- SQL Injection: Prevents malicious database queries.
- Unauthorized Access: Verifies authentication and authorization mechanisms.
- Data Leakage: Ensures sensitive data is not exposed.
Integration Testing
Validates API interactions with external services, databases, and third-party applications to ensure seamless functionality.
Automated Testing Tools
Using automated tools accelerates testing and improves accuracy. Recommended tools include:
- Postman: Simplifies functional and automation testing.
- JUnit: A powerful framework for testing Java-based APIs.
- SoapUI: Ideal for REST and SOAP API testing.
What conclusion can you take from the API Testing?
Deploying and maintaining an API involves careful planning, security considerations, and continuous monitoring. Choosing the right hosting model, implementing an API gateway, and adhering to best practices for versioning and security updates will ensure reliability. Additionally, comprehensive API testing ensures optimal performance and protection against vulnerabilities. By following these best practices, you can build a robust API that meets user expectations while maintaining high availability and security.
Development Tools and Best Practices
APIs (Application Programming Interfaces) are the backbone of modern software development, enabling seamless communication between different systems and applications. Whether you're building a RESTful API or a GraphQL-based service, choosing the right tools and following best practices are essential for efficiency, security, and scalability. In this guide, we explore the top API development tools, best practices, and emerging trends shaping the future of API development.
Top API Development Tools
API Design Tools
Designing an API requires a clear blueprint before implementation.
These tools help developers create, document, and test APIs efficiently:
- Swagger: A widely used tool for designing, documenting, and testing REST APIs.
- Stoplight: A collaborative API design platform with built-in linting and validation features.
- Postman: Originally a testing tool, Postman also supports API design, collaboration, and mock servers.
Frameworks & Libraries
Frameworks and libraries provide the foundation for API development, streamlining routing, authentication, and request handling:
- Express.js (Node.js): A minimal yet powerful framework for building fast and scalable APIs in JavaScript.
- Flask/Django (Python): Flask offers a lightweight and flexible API framework, while Django provides a more opinionated and feature-rich approach.
- Spring Boot (Java): A comprehensive framework that simplifies API development with built-in security, configuration, and scalability options.
API Management Tools
API management tools help organizations control access, monitor performance, and secure their APIs:
- Kong: A cloud-native API gateway with high performance and extensibility.
- Apigee (by Google Cloud): A full-fledged API management platform offering analytics, security, and monetization features.
- AWS API Gateway: A scalable solution for building, deploying, and managing APIs on AWS.
Testing Tools
Testing is crucial for ensuring API reliability and performance. These tools facilitate API testing across various conditions:
- Postman: Automates API testing with support for scripting, mocking, and monitoring.
- JMeter: A load-testing tool used to measure API performance under different traffic conditions.
- SoapUI: Specializes in testing SOAP and REST APIs with advanced features like security testing.
Monitoring & Logging Tools
Monitoring API performance and logging requests are essential for identifying issues and optimizing efficiency:
- New Relic: Provides real-time observability into API performance and error tracking.
- Datadog: A cloud-based monitoring tool offering API metrics, logs, and security insights.
- Prometheus: A popular open-source monitoring system for tracking API health and resource usage.
Which are the Best Practices in API Development?
Following best practices ensures your API is secure, scalable, and user-friendly.
Here are key guidelines:
1. Follow RESTful Principles
Adhering to RESTful principles ensures statelessness and proper use of HTTP methods (GET, POST, PUT, DELETE). This improves consistency and predictability across API endpoints.
2. Use Proper Authentication
Implementing strong authentication mechanisms like OAuth, JWT, or API keys helps secure your API from unauthorized access and potential threats.
3. Implement Caching
Caching API responses using Redis, Memcached, or a CDN reduces server load and improves response times for frequently requested data.
4. Enforce Rate Limiting
Rate limiting prevents abuse by restricting the number of requests a user can make within a given timeframe. This protects API performance and prevents denial-of-service (DoS) attacks.
5. Provide Detailed Error Messages
Using standardized HTTP status codes (e.g., 400 for bad requests, 401 for unauthorized access) and clear error descriptions improves debugging and user experience.
What are the Future Trends in API Development?
API development is constantly evolving, with new trends shaping how APIs are built, managed, and monetized. Here are key trends to watch:
1. GraphQL Growth
GraphQL is becoming a popular alternative to REST due to its flexibility in fetching only the required data. It enables optimized performance and improved developer experience.
2. AI-Driven APIs
The integration of artificial intelligence (AI) and machine learning into APIs is expanding, providing intelligent automation and data-driven insights across industries.
3. Serverless APIs
Serverless architectures, such as AWS Lambda and Google Cloud Functions, enable developers to deploy APIs without managing infrastructure, reducing operational costs and scaling efficiently.
4. API-as-a-Product (API Economy)
APIs are no longer just tools for internal use—they are evolving into standalone business models. Companies are monetizing APIs through subscription-based access, pay-per-use models, and API marketplaces.